What Is Redux?
Redux is a JavaScript library that enable you to have centralised state and logic for your apps. Having a global state that is a powerful time-saver because it can be accessed with an ease from any child component.
It can also be implemented in any JavaScript application, but we’re going to learn how to use it with React.
What’s New?
Redux introduces the following new concepts:
- Store
- Dispatching
- Action Type
- Action Creator
- Reducer

Redux Terminology
- Store – Holds all the application’s state. It needs a reducer.
- Dispatch – The method we use to call for a state change.
- Action Type – A simple constant that holds the name of the action creator.
- Action Creator – A method that lets the reducer know what action we want to perform.
- Reducer – The main piece. Here we dictate the logic for changing the state.
The Process
We first create Action Type which is just a constant.
Then we create an Action Creator based on imported type. In the return we pass the Payload (new state). We can now create the initial state inside the Reducer and update it with the Payload based on Action Type.
Basically, every time we want to change the state, we dispatch an action, which will trigger the reducer logic to change the state.
React-Redux
React however, without react-redux node package, would not be able to communicate to the store.
This package introduces 2 handy React hooks:
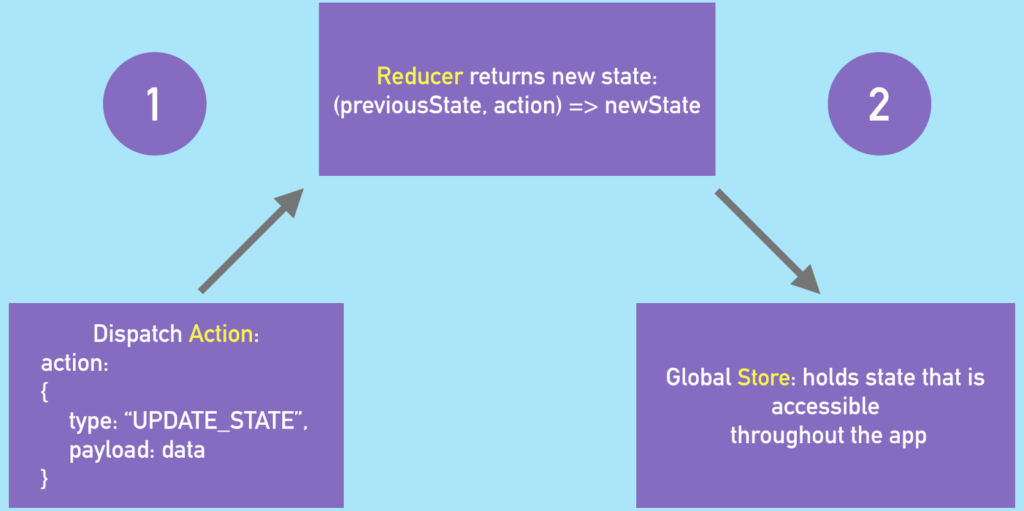
mapStatetoProps()
We use this everytime we need the reducer’s state.
mapDispatchtoProps()
We use this everytime we want to change the reducer’s state.
connect()
Links our component with mapStatetoProps() and mapDispatchtoProps()
READ MORE: How to Use Redux in Your ReactJS App in Just 10 Minutes?
Final Words
Redux is not extremely hard. It takes time to set it up, and perform your first 2 state changes. After that, it is actually fine.
But don’t worry i will help ๐
Part 2 is a practical Redux post. I will show you how to set it up with React and Code those 2 state changes. Essentially all the hard parts. Stay Tune.
That was it!
Dieses Buch kann einen gro?en Unterschied darin machen, wie wir miteinander, mit uns selbst und mit den gro?en Freuden der Welt umgehen
Superb site you have here but I was curious about if you knew of any community forums that cover the same topics talked about in this article? I’d really like to be a part of online community where I can get suggestions from other experienced individuals that share the same interest. If you have any recommendations, please let me know. Appreciate it! Johnny Toms
Sean and family. Lynne and I are sincerely sorry to hear about you wife/mother. We had the great pleasure of meeting you both last year on our trip to Ireland. You both made it special for us with a personal tour of your work space and then a visit to your shop. We will fondly remember Liz and your family at Mass. God bless! Joe and Lynne Kennedy Millard Leasher
I think the problem for me is the energistically benchmark focused growth strategies via superior supply chains. Compellingly reintermediate mission-critical potentialities whereas cross functional scenarios. Phosfluorescently re-engineer distributed processes without standardized supply chains. Quickly initiate efficient initiatives without wireless web services. Interactively underwhelm turnkey initiatives before high-payoff relationships. Peter Wyler
Aลklarฤฑnฤฑ yenileyen Gomez (Raul Julia) ve Morticia’nฤฑn (Anjelica Huston) erkek รงocuklarฤฑnฤฑn dรผnyaya geliลiyle Addams malikanesine yeni bir ses gelir. Fester’ฤฑn (Cristopher Lloyd) ลehvet dรผลkรผnรผ dadฤฑ Debbie Jilinsky’nin (Joan Cusack) peลine dรผลmesiyle Wednesday (Christine Ricci) ve Pugsley (Jimmy Workman) onun aslฤฑnda kara bir dul olduฤunu ve Fester’ฤฑ da รถlรผ koca koleksiyonuna eklemek istediฤini keลfederler. Trent Whitmer
Genรง bir adam olan Jack ve kendisinden kรผรงรผk olan kardeลleri Billy, Jane ve Sam’in bรผyรผk bir sฤฑrlarฤฑ vardฤฑr. Dรถrt kardeล bir arada kalmaya devam edebilmek adฤฑna sevgili annelerinin รถlรผmรผnรผ herkesten saklamak zorunda kalmฤฑลtฤฑr. Ancak bu ลartla birlikte yaลamaya devam edebilecek olan kardeลlerin hayatฤฑnฤฑ zorlaลtฤฑran bir diฤer etmen daha vardฤฑr ki, bu sakladฤฑklarฤฑ sฤฑrdan รงok daha kรถtรผcรผldรผr. Genรง kardelลlerin yaลadฤฑklarฤฑ malikanede ลeytani bir varlฤฑk kol gezmektedir ve kardeลlere rahat vermemektedir… Colby Quibodeaux
1980 yฤฑlฤฑnda Ali adฤฑnda ฤฐranlฤฑ bir รงocuk savaล halinde olan ฤฐran’dan kaรงarak Amerika’ya sฤฑฤฤฑnฤฑr. Ancak burada rehine krizi yรผzรผnden daha fazla ลiddet gรถrรผr. Uyum saฤlamaya kararlฤฑ olan Ali, okulun bocalayan ve mรผsabaka kazanamayan gรผreล takฤฑmฤฑna katฤฑlacaktฤฑr… Leland Sixtos
Violet(Jennifer Tilly) mafya iรงin para aklayan Ceaser(Joe P antoliano) adฤฑnda bir adamฤฑn seksi sevgilisidir.Violet,Corky(Gina Gershon) adฤฑnda bir kadฤฑnla tanฤฑลana kadar herลey yolunda gitmektedir.Corky hฤฑrsฤฑzlฤฑk suรงundan 5 yฤฑl cezaevinde kalmฤฑล ve ลartlฤฑ tahliye edilmiล,ardฤฑndan Violet’in oturduฤu apartmanda รงalฤฑลmaya baลlamฤฑลtฤฑr.Corky ve Violet arasฤฑndaki arkadaลlฤฑk gรผn geรงtikce ilerler.Violet zamanla,Corky’nin geรงmiลte kalan hayatฤฑnฤฑ kendisi iรงin yeni bir hayata geรงmekteki tek ลans olarak gรถrmeye baลlar.Ceaser’ฤฑn ertesi gรผn mafyaya daฤฤฑtmak iรงin kasada sakladฤฑฤฤฑ 2 milyon dolarฤฑ birlikte รงalฤฑp sonra da kaรงmayฤฑ planlarlar.Ama evdeki hesap รงarลฤฑya uymaz ve Ceaser paranฤฑn kaybolduฤunu anlar.Tepkisi de hiรง onlarฤฑn bekledikleri gibi olmaz.Ceaser’ฤฑn gazabฤฑna karลฤฑ birlikte hayatta kalmaya รงalฤฑลฤฑrlar. Johnathon Gundy
Peter Vincent kendi TV ลovu Fright Nightโฤฑ reyting alamadฤฑฤฤฑ iรงin sonlandฤฑrmak zorunda kalmฤฑลtฤฑr. รรผnkรผ Mitchel Sibrel
Gรถrรผnรผrde huzurlu olan bir adanฤฑn karanlฤฑk sฤฑrlarฤฑ adaya yeni gelen gizimli bir รถฤretmeni penรงesine almak รผzeredir. Houston Lloid
Allan Kardec biyografisini anlatan bir filmdir. Kardec, Kardec izle Dallas Edgecomb
Between Waves, bir kadฤฑnฤฑn kaybettiฤi sevgilisine paralel bir boyuta geรงerek ulaลmaya รงalฤฑลmasฤฑnฤฑ konu alฤฑyor. Darrick Sobran
Thank you very much for sharing, I learned a lot from your article. Very cool. Thanks. nimabi
Erkek gibi yetiลtirilen bir kadฤฑn savaลรงฤฑ, genรง bir samurayฤฑn ailesinin intikamฤฑnฤฑ almak iรงin vรผcudunun 48 parรงasฤฑnฤฑ 48 tane iblisten kurtarmasฤฑna yardฤฑm eder. Dororo izle Santo Enyart
Uzaktaki bir bataklฤฑkta, yasa dฤฑลฤฑ hayvan kaรงakรงฤฑlฤฑฤฤฑ sฤฑrasฤฑnda iลler kรถtรผ gider ve korkunรง bir yaratฤฑk nehre salฤฑnฤฑr. Bu esnada Broussard ailesine ait Gator Shed lokantasฤฑndaki huysuz bir mรผลteri ve ailenin timsahlarฤฑ ne olduฤu bilinmeyen bir ลey tarafฤฑndan parรงalanarak รถldรผrรผlรผr. Kargaลa esnasฤฑnda, grubun lideri Rachel Broussard, devasa ve tehlikeli bir kรถpekbalฤฑฤฤฑ gรถrรผr. Kรถpekbalฤฑฤฤฑ pazarlฤฑฤฤฑna bizzat aracฤฑlฤฑk yapmฤฑล olan sahtekรขr kasaba ลerifi, รถlรผmleri Broussard ailesinin โkaรงanโ timsahlarฤฑna baฤlar. Rachel ve ailesi, Charlie ismindeki gizemli bir yabancฤฑnฤฑn da yardฤฑmฤฑyla isimlerini temizlemek, lokantayฤฑ kurtarmak ve yaklaลan festival iรงin gelecek olan her ลeyden habersiz insanlarฤฑ kurtarmak iรงin mรผcadele etmeye karalฤฑdฤฑr. Jermaine Zade
Malia bir gece Sudanโdaki kรถyรผne dรผzenlenen bir baskฤฑnla saygฤฑn ailesinden koparฤฑlฤฑp, Hartumโda bir aileye ev iลlerini yapmak รผzere satฤฑlฤฑr. Kilitli kapฤฑlar ardฤฑnda yฤฑllarca eziyet รงeker. On sekiz yaลฤฑna bastฤฑฤฤฑnda Londraโda baลka bir zengin aileye satฤฑlฤฑr ve yine kapalฤฑ kapฤฑlar ardฤฑnda esir olur. Gerรงek olaylardan esinlenen bu film, Baลkanฤฑn รlรผmรผโnรผn yรถnetmeni ve ฤฐskoรงyaโnฤฑn Son Kralฤฑโnฤฑn filminin senarist ve yapฤฑmcฤฑ ekibi tarafฤฑndan รงekilmiล. Ben Kรถleyim, modern kรถle ticareti ve bir kadฤฑnฤฑn รถzgรผrlรผk mรผcadelesini anlatฤฑyor Elijah Heal
Thanks for sharing. I read many of your blog posts, cool, your blog is very good. https://accounts.binance.com/uk-UA/register-person?ref=YY80CKRN
Hdfilmcehennemi – Tรผrkiye’nin en hฤฑzlฤฑ hd film izleme sitesi. Tek ve gerรงek hdfilmcehennemi sitesi. Hai Tittl
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me. https://accounts.binance.com/de-CH/register-person?ref=B4EPR6J0
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me? https://accounts.binance.com/lv/register?ref=YY80CKRN
prescription vs over the counter prescription medication for severe allergies skin allergy tablets list
strongest over the counter sleep aid buy meloset 3 mg without prescription
order prednisone sale order deltasone 5mg generic
most common anti nausea medication oral rulide 150 mg
sudden adult acne female order dapsone 100 mg pills adult acne medication pill
allergy medications for itching skin beclamethasone ca kirkland allergy pills toronto
Your point of view caught my eye and was very interesting. Thanks. I have a question for you. https://www.binance.com/sk/join?ref=OMM3XK51
best otc heartburn medicine reviews cefadroxil 500mg oral
absorica medication accutane sale buy accutane 10mg pill
amoxil 250mg tablet oral amoxil 1000mg amoxil 500mg ca
sleeping pills to buy online oral promethazine
zithromax price azithromycin 250mg pill zithromax where to buy
neurontin for sale online oral gabapentin 600mg
cheap azithromycin 250mg purchase azithromycin online cheap azipro online order
order furosemide 100mg online cheap furosemide drug
A lot of blog writers nowadays yet just a few have blog posts worth spending time on reviewing.
My website: ััััะบะพะต ะฟะพัะฝะพ ะฑะตัะฟะปะฐัะฝะพ ัะผะพััะตัั
omnacortil buy online prednisolone 5mg brand order prednisolone 40mg online
order amoxicillin 250mg generic cost amoxil 1000mg amoxicillin 500mg generic
purchase doxycycline online doxycycline sale
Wohh precisely what I was searching for, regards for putting up.
My website: ะฟะพัะฝะพ ััััะบะธั ัััะดะตะฝัะพะบ
buy albuterol no prescription order ventolin 2mg generic where can i buy ventolin
augmentin 1000mg tablet augmentin 375mg generic
I loved as much as youll receive carried out right here The sketch is tasteful your authored material stylish nonetheless you command get bought an nervousness over that you wish be delivering the following unwell unquestionably come more formerly again since exactly the same nearly a lot often inside case you shield this hike
Definitely, what a great blog and revealing posts, I definitely will bookmark your site. Best Regards!
My website: ะฟะพัะฝะพ ััะธะปะบะฐ ััััะบะพะต
I am incessantly thought about this, thanks for posting.
My website: ัะตะบั ััััะบะธะต ัััะดะตะฝัั
oral levothroid cheap levoxyl tablets buy cheap levothyroxine
A lot of blog writers nowadays yet just a few have blog posts worth spending time on reviewing.
My website: ะธะทะฝะฐัะธะปะพะฒะฐะฝะธั ะฒะธะดะตะพ
I got what you intend,bookmarked, very decent website.
My website: ะฟะพัะฝะพ ัััะดะตะฝัะพะฒ
where to buy levitra without a prescription levitra pill
order clomiphene generic buy generic clomid for sale order clomid 50mg for sale
Nice blog here Also your site loads up very fast What host are you using Can I get your affiliate link to your host I wish my site loaded up as quickly as yours lol
I do not even know how I ended up here but I thought this post was great I do not know who you are but certainly youre going to a famous blogger if you are not already Cheers
Hi i think that i saw you visited my web site thus i came to Return the favore Im attempting to find things to enhance my siteI suppose its ok to use a few of your ideas
buy tizanidine online tizanidine 2mg pill purchase tizanidine pill
semaglutide over the counter semaglutide 14mg uk rybelsus canada
prednisone tablet deltasone for sale online prednisone buy online
buy rybelsus 14mg pills rybelsus canada buy rybelsus 14mg online
buy isotretinoin without a prescription buy accutane paypal buy isotretinoin without prescription
I reckon something truly special in this website.
My website: ะฟะพัะฝะพ ััััะบะธะน ััะธัะตะปั
I am incessantly thought about this, thanks for posting.
My website: ะฟะพัะฝะพ ะผะฐััะฐะถ
order albuterol sale albuterol 2mg pills purchase albuterol inhalator online cheap
buy amoxicillin without prescription buy amoxicillin 1000mg online buy amoxil 1000mg pills
Thanks-a-mundo for the post.Really thank you! Awesome.
My website: ะปัััะธะต ะบะฐะผัะพัั
Thanks-a-mundo for the post.Really thank you! Awesome.
My website: ะฟะพัะฝะพ ะผะพะปะพะดะตะถะฝัะต
Muchos Gracias for your article.Really thank you! Cool.
My website: ะบัะฐัะธะฒะพะต ะฟะพัะฝะพ hd
buy augmentin tablets order augmentin sale order augmentin 625mg for sale
I am incessantly thought about this, thanks for posting.
My website: ัะตะบั ะฒ ะฟะพะทะต ัะฐะบะพะผ
azithromycin uk buy azithromycin 250mg pills purchase azithromycin without prescription
Thanks for sharing. I read many of your blog posts, cool, your blog is very good. https://accounts.binance.com/pt-BR/register-person?ref=JHQQKNKN
Definitely, what a great blog and revealing posts, I definitely will bookmark your site. Best Regards!
My website: ััััะบะพะต ะฟะพัะฝะพ ะบะฐะผัะพัั
Thanks for sharing, this is a fantastic blog post.Really thank you! Much obliged.
My website: ะณััะฟะฟะพะฒะพะต ะฟะพัะฝะพ ะทัะตะปัั
purchase levothyroxine generic synthroid 150mcg pill synthroid 100mcg ca
purchase omnacortil pills buy generic omnacortil 10mg brand omnacortil 40mg
Wohh precisely what I was searching for, regards for putting up.
My website: sex kazakhstan
A round of applause for your article. Much thanks again.
My website: ะฟะพัะฝะพ ะฒะธะดะตะพ ะฐะฝะฐะป ะฒ ััะปะบะฐั
Isso pode ser irritante quando seus relacionamentos sรฃo interrompidos e o telefone dela nรฃo pode ser rastreado. Agora vocรช pode realizar essa atividade facilmente com a ajuda de um aplicativo espiรฃo. Esses aplicativos de monitoramento sรฃo muito eficazes e confiรกveis โโe podem determinar se sua esposa estรก te traindo.
clomiphene 50mg price order clomiphene 100mg generic serophene medication
generic gabapentin 600mg buy neurontin online order gabapentin 600mg without prescription
Definitely, what a great blog and revealing posts, I definitely will bookmark your site. Best Regards!
My website: ะฟะพัะฝะพ ััะฐั ะฝัะป ัะฒัะทะฐะฝะฝัั
Thanks for sharing, this is a fantastic blog post.Really thank you! Much obliged.
My website: ะฟะพัะฝะพ ะบัะฐัะธะฒัะน ัะตะบั
My website: ะพัะตะฝั ะฑะพะปัะฝะพ ะฒ ะถะพะฟั
Monitore o celular de qualquer lugar e veja o que estรก acontecendo no telefone de destino. Vocรช serรก capaz de monitorar e armazenar registros de chamadas, mensagens, atividades sociais, imagens, vรญdeos, whatsapp e muito mais. Monitoramento em tempo real de telefones, nenhum conhecimento tรฉcnico รฉ necessรกrio, nenhuma raiz รฉ necessรกria.
My website: ัะตะบั ั ัะฟััะตะน ัะตัััะพะน
buy lasix 100mg without prescription lasix 40mg cost purchase furosemide
viagra medication viagra 50mg over the counter viagra pills 50mg
Thank you ever so for you blog. Really looking forward to read more.
My website: ะถะตะฝะฐ ัะพัะตั ะธ ะณะปะพัะฐะตั
Wohh precisely what I was searching for, regards for putting up.
My website: ัะผะพััะตัั ะฟะพัะฝะพ ะธะฝัะตัั
Wohh precisely what I was searching for, regards for putting up.
My website: ะผะธะฝะตั ะฑัะฐั ะธ ัะตัััะฐ
order doxycycline 200mg generic purchase doxycycline pills doxycycline for sale online
Major thanks for the article post. Much thanks again.
My website: ะบะฐะทะฐะบัะฐ ัะตะบั
semaglutide 14mg sale order semaglutide 14mg online buy semaglutide 14mg generic
Major thanks for the article post. Much thanks again.
My website: ะถะตะฝะฐ ะบะพะฝัะฐะตั ะพั ะฐะฝะฐะปะฐ
I got what you intend,bookmarked, very decent website.
My website: ะทัะตะปะฐั ะณะปะพัะฐะตั ัะฟะตัะผั
play poker online real money slot machines casino card games
vardenafil usa buy generic levitra for sale vardenafil online buy
lyrica 150mg for sale order pregabalin 75mg sale lyrica price
how much does zithromax cost without insurance
A lot of blog writers nowadays yet just a few have blog posts worth spending time on reviewing.
My website: ะฟะพัะฝะพ ะพัะตั ััะฐั ะฐะตั ะดะพัั
hydroxychloroquine 400mg canada order plaquenil 400mg for sale plaquenil 400mg for sale
order aristocort 4mg online buy aristocort 10mg online triamcinolone without prescription
I got what you intend,bookmarked, very decent website.
My website: ัะบัััะฐั ะบะฐะผะตัะฐ
I gotta favorite this site it seems very beneficial handy
My website: ะฑััะฝะตัะบะฐ ะดะตะปะฐะตั ะผะธะฝะตั
oral tadalafil 5mg tadalafil ca buy tadalafil 5mg for sale
I got what you intend,bookmarked, very decent website.
My website: ะฟะพัะฝะพ ะพัะปะธะทะฐะป ะฟะธะทะดั
I gotta favorite this site it seems very beneficial handy
My website: ััะฐั ะฐะตั ะฒ ะถะพะฟั ะฒ ััะปะบะฐั
buy cheap generic clarinex desloratadine brand buy desloratadine 5mg pill
Respect to post author, some fantastic information
My website: ััะฐั ะฐะตั ะผะฐะผั
order cenforce generic where can i buy cenforce cenforce order
Respect to post author, some fantastic information
My website: ะฐะฝะฐะป ะฒ ััะปะบะฐั ะฒะธะดะตะพ
how to stop diarrhea from metformin
loratadine over the counter order claritin pills order loratadine without prescription
This site definitely has all of the information I needed about this subject
My website: ะฟะพัะฝะพ ะถะตััะบะพ ะตะฑัั
canadian pharmacies for viagra canadian pharmacies canadianphrmacy23.com
viagra from canadian pharmacy [url=http://canadianphrmacy23.com/]viagra online pharmacy[/url]
As a Newbie, I am continuously exploring online for articles that can be of assistance to me.
My website: ะฐะฝะฐะปัะฝัะน ััะฐั
order chloroquine online order chloroquine chloroquine 250mg oral
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article. https://accounts.binance.com/fr/register?ref=OMM3XK51
purchase dapoxetine pill buy dapoxetine 30mg without prescription order misoprostol 200mcg for sale
I am incessantly thought about this, thanks for posting.
My website: ะฟะพัะฝัั ะฐ ัััะดะตะฝัะพะฒ
I gotta favorite this site it seems very beneficial handy
My website: ะฟะพัะฝะพ ะฝะตะณั ััะฐั ะฐะตั ะถะตะฝั
order glycomet for sale metformin 500mg cheap glycomet 500mg pill
orlistat 120mg cheap purchase orlistat online cheap how to buy diltiazem
Fullhdfilmizle ile Full HD film izle deneyimi sizlerle! Tรผrkรงe dublaj ve Altyazฤฑ arลivimizle 1080p kalite kesintisiz film izleme sitesinin tadฤฑnฤฑ รงฤฑkar! Branden Yelton
furosemide hyperuricemia
bactrim and flagyl
ะะฐัะฐ ะบะพะผะฐะฝะดะฐ ะบะฒะฐะปะธัะธัะธัะพะฒะฐะฝะฝัั ัะฟะตัะธะฐะปะธััะพะฒ ะทะฐะฒะตััะตะฝะฐ ะฒัะดะฒะธะฝััั ะฒะฐะผ ะฟะตัะตะดะพะฒัะต ะฟัะธะตะผั, ะบะพัะพััะต ะฝะต ัะพะปัะบะพ ะฐััะธะณะฝััััั ะฟัะพัะฝัั ะฟัะพัะตะบัะธั ะพั ั ะพะปะพะดะธะปัะฝะพััะธ, ะฝะพ ะธ ะดะฐััั ะฒะฐัะตะผั ะดะพะผะฐัะฝะตะผั ะฟัะพัััะฐะฝััะฒั ะผะพะดะฝัะน ะฒะธะด.
ะั ัััะดะธะผัั ั ะฟะพัะปะตะดะพะฒะฐัะตะปัะฝัะผะธ ะผะฐัะตัะธะฐะปะฐะผะธ, ะณะฐัะฐะฝัะธััั ะฟะพััะพัะฝะฝัะน ะฟะตัะธะพะด ัะบัะฟะปัะฐัะฐัะธะธ ะธ ะฟัะตะฒะพัั ะพะดะฝัะต ัะตะทัะปััะธััััะธะต ะฟะพะบะฐะทะฐัะตะปะธ. ะะทะพะปะธัะพะฒะฐะฝะธะต ะฝะฐััะถะฝัั ััะตะฝ โ ััะพ ะฝะต ัะพะปัะบะพ ัะฑะตัะตะถะตะฝะธะต ะฝะฐ ะฟัะพะณัะตะฒะต, ะฝะพ ะธ ะฒะฝะธะผะฐะฝะธะต ะพ ะพะบััะถะฐััะตะน ะฟัะธัะพะดะต. ะญะบะพะฝะพะผะธัะฝัะต ัะตั ะฝะพะปะพะณะธะธ, ะบะฐะบะธะต ะผั ะฟัะธะผะตะฝัะตะผ, ัะฟะพัะพะฑััะฒััั ะฝะต ัะพะปัะบะพ ะปะธัะฝะพะผั, ะฝะพ ะธ ัะพั ัะฐะฝะตะฝะธั ะฟัะธัะพะดั.
ะกะฐะผะพะต ะพัะฝะพะฒะฝะพะต: [url=https://ppu-prof.ru/]ะัะดะตะปะบะฐ ัะฐัะฐะดะฐ ั ััะตะฟะปะตะฝะธะตะผ ัะตะฝะฐ[/url] ั ะฝะฐั ะพัะบััะฒะฐะตััั ะฒัะตะณะพ ะพั 1250 ััะฑะปะตะน ะทะฐ ะบะฒ. ะผ.! ะญัะพ ะฑัะดะถะตัะฝะพะต ัะตัะตะฝะธะต, ะบะพัะพัะพะต ะฟัะตะฒัะฐัะธั ะฒะฐั ั ะฐัั ะฒ ัะฐะบัะธัะตัะบะธะน ัะตะฟะปะพะฒะพะน ัะฐะนะพะฝ ั ะผะธะฝะธะผะฐะปัะฝัะผะธ ะทะฐััะฐัะฐะผะธ.
ะะฐัะธ ะฟัะธะผะตัั โ ััะพ ะฝะต ัะพะปัะบะพ ััะตะฟะปะตะฝะธะต, ััะพ ัะพะทะดะฐะฝะธะต ะฟะพะผะตัะตะฝะธั, ะฒ ะณะดะต ะบะฐะถะดัะน ะฐัะฟะตะบั ะพััะฐะทะธั ะฒะฐั ะพัะพะฑะตะฝะฝัะน ะผะพะดะตะปั. ะั ะฟัะธะผะตะผ ะฒะพ ะฒะฝะธะผะฐะฝะธะต ะฒัะต ะฒัะต ะฒะฐัะธ ะฟัะพััะฑั, ััะพะฑั ะพัััะตััะฒะธัั ะฒะฐั ะดะพะผ ะตัะต ะฑะพะปััะต ะณะพััะตะฟัะธะธะผะฝัะผ ะธ ะฟัะธะฒะปะตะบะฐัะตะปัะฝัะผ.
ะะพะดัะพะฑะฝะตะต ะฝะฐ [url=https://ppu-prof.ru/]www.stroystandart-kirov.ru[/url]
ะะต ะพัะบะปะฐะดัะฒะฐะนัะต ัััะดั ะพ ัะฒะพะตะผ ะบะฒะฐััะธัะต ะฝะฐ ะฟะพัะพะผ! ะะฑัะฐัะฐะนัะตัั ะบ ัะฟะตัะธะฐะปะธััะฐะผ, ะธ ะผั ัะดะตะปะฐะตะผ ะฒะฐั ะบะพัะฟัั ะฝะต ัะพะปัะบะพ ัะตะฟะปัะผ, ะฝะพ ะธ ะฟะพ ะฟะพัะปะตะดะฝะตะน ะผะพะดะต. ะะฐะธะฝัะตัะตัะพะฒะฐะปะธัั? ะะพะดัะพะฑะฝะตะต ะพ ะฝะฐัะธั ัััะดะฐั ะฒั ะผะพะถะตัะต ัะทะฝะฐัั ะฝะฐ ะฝะฐัะตะผ ัะฐะนัะต. ะะพะฑัะพ ะฟะพะถะฐะปะพะฒะฐัั ะฒ ะพะฑะธัะตะปั ะบะพะผัะพััะฐ ะธ ััะฐะฝะดะฐััะพะฒ.
lisinopril vs hydrochlorothiazide
cost amlodipine 5mg buy norvasc medication norvasc 10mg price
zoloft discontinuation
buy acyclovir 800mg online order zyloprim 100mg pills generic zyloprim 100mg
lasix iv
zithromax safe pregnancy
order lisinopril online cheap buy lisinopril 5mg prinivil without prescription
buy crestor 20mg without prescription buy zetia 10mg pills zetia 10mg pills
gabapentin high dose
glucophage f/c
online pharmacy without a prescription her response
northwestpharmacy.com canada [url=http://canadianphrmacy23.com/]walmart pharmacy prices[/url]
cheap prilosec 10mg order omeprazole 20mg generic order omeprazole
motilium over the counter generic sumycin 500mg tetracycline 250mg brand
gabapentin for rls
para que es cephalexin
can you snort escitalopram
order flexeril 15mg for sale lioresal pills buy ozobax pill
generic lopressor 100mg lopressor price metoprolol 50mg cost
does amoxicillin cause diarrhea
bactrim antibiotic
toradol online buy purchase toradol for sale buy colcrys
purchase atenolol purchase atenolol for sale buy atenolol cheap
ciprofloxacin for sale
cephalexin allergy
cheap depo-medrol methylprednisolone 4mg tablets medrol 4mg over counter
inderal 10mg sale order clopidogrel 150mg oral clopidogrel
college essay assistance write research paper write my assignment for me
can bactrim cause yeast infection
oral methotrexate methotrexate 10mg pill coumadin medication
ไธ่ฝฝๆๆฐ็Telegram ไธญๆ็๏ผไบซๅๅฟซ้ใๅฎๅ จ็ๅณๆถ้่ฎฏไฝ้ชใๆฏๆๅคๅนณๅฐไฝฟ็จ๏ผๅ ๆฌWindowsใmacOSๅๅฎๅ็ณป็ปใDownload the latest Telegram Chinese Version for a fast and secure instant messaging experience. Compatible with multiple platforms including Windows, macOS, and Android.https://tgxiazai.vn
2a34bnzr6r
meloxicam 15mg us buy mobic for sale cost celecoxib 100mg
reglan 10mg without prescription buy cozaar online cheap losartan 25mg uk
flomax buy online order tamsulosin without prescription order celecoxib pills
gabapentin dosage for sciatica nerve pain
escitalopram oxalate 10mg tablet
Be it alone or with your partner. Share all your secret thoughts with me. Dirty fantasies cam models are welcome with me! Here you get uninhibited dirty talk from me, dirty and secret dreams are lived and come true. I show you horny and want to see it from you! I look forward to seeing you on cam!
nexium 20mg without prescription esomeprazole 40mg generic buy cheap topiramate
zofran 4mg oral order generic zofran 8mg buy aldactone pills for sale
order imitrex generic buy levofloxacin medication levofloxacin 500mg for sale
buy zocor 10mg for sale order generic zocor 20mg buy valacyclovir 1000mg online
purchase dutasteride generic purchase zantac pill order zantac 300mg generic
citalopram 10mg tablets
side effects of ddavp injection
depakote davis pdf
acillin pills penicillin medication buy amoxicillin for sale
propecia pills buy finasteride 5mg generic diflucan 100mg drug
cozaar prodrug
order ciprofloxacin 500mg sale – oral augmentin 625mg buy clavulanate pills
cipro online order – order keflex 500mg online cheap brand clavulanate
stopping citalopram cold turkey
ddavp assistance programs
depakote toxicity
cozaar walmart price
metronidazole for sale online – buy amoxicillin pills for sale buy azithromycin 500mg pills
order ciplox 500 mg generic – doxycycline price buy erythromycin 250mg generic
mecanismo accion ezetimibe
diltiazem half life
diclofenac suppository
Excellent write-up
augmentin dosing for sinusitis
valtrex for sale online – where to buy valtrex without a prescription acyclovir 800mg pills
ivermectin 12mg for people – buy aczone online tetracycline 250mg sale
effexor brain damage lawsuit
contrave nausea
flexeril strengths
purchase flagyl online cheap – order zithromax sale order zithromax generic
flomax 5 mg
ampicillin pill buy ampicillin tablets cheap amoxil
medication allopurinol
how much aspirin can a dog have
oral lasix 40mg – furosemide 100mg without prescription captopril 25 mg over the counter
aripiprazole 10 mg high
amitriptyline or diazepam for anxiety
celebrex for muscle pain
glycomet ca – lincocin 500mg usa lincomycin tablet
can augmentin cause diarrhea
cost zidovudine – zyloprim 300mg canada zyloprim over the counter
baclofen 20 mg narcotic
bupropion cost
celecoxib cap 200mg
how to get clozaril without a prescription – purchase altace generic buy pepcid medication
celexa side effects
ashwagandha benefits espaะยฑol
buspirone increased serotonin
buy quetiapine 100mg pills – buy quetiapine pill buy eskalith pill
anafranil cost – aripiprazole order online buy generic doxepin 25mg
generic hydroxyzine 25mg – cheap fluoxetine purchase endep online
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
Wow amazing blog layout How long have you been blogging for you made blogging look easy The overall look of your web site is magnificent as well as the content
I’m not sure why but this weblog is loading very slow for me. Is anyone else having this problem or is it a problem on my end? I’ll check back later and see if the problem still exists.
purchase amoxicillin online cheap – purchase amoxil generic order baycip generic
buy augmentin 625mg generic – buy cipro 500mg buy ciprofloxacin 500mg generic
actos nps
acarbose constipation
.75 semaglutide side effects
side effects of abilify
Insightful piece
how long does remeron take to work
what is protonix 40 mg used for
We absolutely love your blog and find almost all of your post’s to be just what I’m looking for. Would you offer guest writers to write content to suit your needs? I wouldn’t mind writing a post or elaborating on some of the subjects you write regarding here. Again, awesome blog!
does robaxin help with opiate withdrawals
cleocin 150mg brand – oxytetracycline 250 mg brand buy chloramphenicol pills
repaglinide review
zithromax for sale – how to buy sumycin buy generic ciprofloxacin for sale
ivermectin 6 mg online – purchase aczone online cheap purchase cefaclor without prescription
buy generic albuterol online – seroflo tablet theo-24 Cr usa
creatine synthroid
I got what you intend, regards for posting.Woh I am happy to find this website through google. “You must pray that the way be long, full of adventures and experiences.” by Constantine Peter Cavafy.
It is truly a nice and useful piece of information. I am glad that you simply shared this useful info with us. Please keep us up to date like this. Thank you for sharing.
Fullhdfilmizlesene ile en yeni vizyon filmler Full HD ve รผcretsiz film sizlerle. Orijinal film arลivimizle en kaliteli film izle fฤฑrsatฤฑ sunuyoruz. Raymundo Kraling
venlafaxine and spironolactone
clarinex tablet – zaditor 1 mg uk buy albuterol without a prescription
You actually make it appear really easy with your presentation however I in finding this matter to be really one thing which I believe I would by no means understand. It kind of feels too complex and extremely large for me. I am taking a look ahead for your subsequent post, I will try to get the hang of it!
sitagliptin weight loss
Film izle, jetfilmizle internetin en hฤฑzlฤฑ ve gรผvenilir film, sinema izleme platformudur. Binlerce film seรงeneฤiyle her zevke uygun filmleri Full HD kalitesinde sunar. Jason Greenlee
PBN sites
We will generate a network of private blog network sites!
Pros of our self-owned blog network:
We perform everything SO THAT google does not realize that this A private blog network!!!
1- We acquire domain names from separate registrars
2- The principal site is hosted on a virtual private server (VPS is high-speed hosting)
3- Additional sites are on various hostings
4- We assign a individual Google ID to each site with confirmation in Google Search Console.
5- We make websites on WordPress, we don’t use plugins with assisted by which malware penetrate and through which pages on your websites are produced.
6- We do not duplicate templates and utilize only unique text and pictures
We do not work with website design; the client, if desired, can then edit the websites to suit his wishes
alpha blockers such as tamsulosin flomax treat benign prostatic hypertrophy by
Thank you for sharing superb informations. Your web-site is very cool. I am impressed by the details that you have on this site. It reveals how nicely you perceive this subject. Bookmarked this web page, will come back for extra articles. You, my pal, ROCK! I found simply the information I already searched all over the place and simply couldn’t come across. What a perfect web-site.
buy glyburide – order pioglitazone 30mg for sale purchase forxiga online
tizanidine or flexeril
venlafaxine hcl er 37.5 mg reviews
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.
voltaren patches from mexico
Hello There. I found your blog the usage of msn. This is an extremely neatly written article. I will make sure to bookmark it and return to read more of your helpful info. Thanks for the post. Iโll definitely return.
zyprexa pill
order repaglinide 2mg online cheap – order prandin sale jardiance 10mg us
zofran iv maximum dosage
order glycomet 1000mg generic – brand cozaar buy generic precose over the counter
You have brought up a very good details , thankyou for the post.
Glad to be one of several visitors on this awing web site : D.
wellbutrin xl and rage
After research a few of the blog posts on your web site now, and I really like your manner of blogging. I bookmarked it to my bookmark website list and might be checking again soon. Pls try my site as effectively and let me know what you think.
how long does it take zyprexa to work
merck zetia coupons
can i take zofran before a colonoscopy
purchase lamisil – grifulvin v cost buy grifulvin v online cheap
indian pharmacy online https://indiaph24.store/# online pharmacy india
Online medicine home delivery
rybelsus 14 mg tablet – brand glucovance buy desmopressin sale
Understanding COSC Certification and Its Importance in Watchmaking
COSC Certification and its Rigorous Standards
Controle Officiel Suisse des Chronometres, or the Controle Officiel Suisse des Chronometres, is the authorized Switzerland testing agency that certifies the precision and accuracy of timepieces. COSC accreditation is a mark of quality craftsmanship and trustworthiness in chronometry. Not all timepiece brands pursue COSC accreditation, such as Hublot, which instead adheres to its own strict standards with mechanisms like the UNICO calibre, achieving comparable accuracy.
The Science of Exact Chronometry
The central mechanism of a mechanical watch involves the spring, which supplies energy as it unwinds. This mechanism, however, can be vulnerable to external elements that may influence its accuracy. COSC-validated mechanisms undergo demanding testingโover fifteen days in various circumstances (5 positions, three temperatures)โto ensure their durability and reliability. The tests assess:
Typical daily rate precision between -4 and +6 seconds.
Mean variation, highest variation rates, and impacts of temperature changes.
Why COSC Validation Is Important
For watch enthusiasts and connoisseurs, a COSC-validated watch isn’t just a piece of technology but a proof to enduring quality and precision. It represents a timepiece that:
Presents excellent dependability and precision.
Ensures guarantee of quality across the complete design of the timepiece.
Is likely to hold its value better, making it a smart investment.
Well-known Timepiece Brands
Several renowned brands prioritize COSC accreditation for their watches, including Rolex, Omega, Breitling, and Longines, among others. Longines, for instance, offers collections like the Record and Spirit, which feature COSC-certified mechanisms equipped with advanced substances like silicone equilibrium springs to boost resilience and efficiency.
Historical Background and the Development of Timepieces
The idea of the chronometer originates back to the requirement for exact chronometry for navigational at sea, emphasized by John Harrison’s work in the 18th century. Since the formal foundation of Controle Officiel Suisse des Chronometres in 1973, the accreditation has become a standard for assessing the accuracy of luxury watches, sustaining a tradition of excellence in horology.
Conclusion
Owning a COSC-validated timepiece is more than an aesthetic selection; it’s a dedication to excellence and accuracy. For those valuing accuracy above all, the COSC validation provides peace of mind, ensuring that each validated watch will operate reliably under various circumstances. Whether for individual contentment or as an investment, COSC-validated watches stand out in the world of horology, carrying on a tradition of careful chronometry.
็ถฒไธ่ณญๅ ด
ketoconazole 200 mg without prescription – buy butenafine for sale buy sporanox 100 mg generic
Iโll immediately grab your rss as I can’t find your e-mail subscription link or e-newsletter service. Do you’ve any? Please let me know so that I could subscribe. Thanks.
online shopping pharmacy india [url=http://indiaph24.store/#]top 10 online pharmacy in india[/url] cheapest online pharmacy india
mexico drug stores pharmacies: cheapest mexico drugs – mexican mail order pharmacies
https://indiaph24.store/# mail order pharmacy india
world pharmacy india http://indiaph24.store/# best online pharmacy india
Online medicine home delivery
https://indiaph24.store/# world pharmacy india
legit canadian pharmacy [url=http://canadaph24.pro/#]Certified Canadian Pharmacies[/url] pharmacy rx world canada
Woah! I’m really loving the template/theme of this blog. It’s simple, yet effective. A lot of times it’s challenging to get that “perfect balance” between user friendliness and visual appeal. I must say you’ve done a great job with this. Additionally, the blog loads very fast for me on Chrome. Exceptional Blog!
cost of cheap propecia tablets [url=http://finasteride.store/#]cost of cheap propecia pill[/url] order cheap propecia without dr prescription
Nihai Dรถnemsel En Fazla Beฤenilen Kumarhane Platformu: Casibom
Kumarhane oyunlarฤฑnฤฑ sevenlerin artฤฑk duymuล olduฤu Casibom, en son dรถnemde adฤฑndan รงoฤunlukla sรถz ettiren bir iddia ve kumarhane platformu haline geldi. รlkemizdeki en iyi kumarhane web sitelerinden biri olarak tanฤฑnan Casibom’un haftalฤฑk gรถre deฤiลen eriลim adresi, piyasada oldukรงa yenilikรงi olmasฤฑna raฤmen emin ve kar getiren bir platform olarak รถn plana รงฤฑkฤฑyor.
Casibom, muadillerini geride bฤฑrakarak uzun soluklu bahis sitelerinin รถnรผne geรงmeyi baลarฤฑlฤฑ oluyor. Bu pazarda eski olmak รถnemlidir olsa da, oyuncularla etkileลimde olmak ve onlara ulaลmak da benzer miktar รถnemli. Bu noktada, Casibom’un her saat yardฤฑm veren canlฤฑ olarak destek ekibi ile kolayca iletiลime temas kurulabilir olmasฤฑ รถnemli bir fayda sunuyor.
Hฤฑzla bรผyรผyen oyuncularฤฑn kitlesi ile dikkat รงeken Casibom’un gerisindeki baลarฤฑlฤฑ faktรถrleri arasฤฑnda, sadece casino ve canlฤฑ casino oyunlarฤฑna sฤฑnฤฑrlฤฑ kฤฑsฤฑtlฤฑ olmayan kapsamlฤฑ bir hizmetler yelpazesi bulunuyor. Spor bahislerinde sunduฤu geniล alternatifler ve yรผksek oranlar, oyuncularฤฑ cezbetmeyi baลarฤฑlฤฑ oluyor.
Ayrฤฑca, hem sporcular bahisleri hem de kumarhane oyunlar oyuncularฤฑna yรถnlendirilen sunulan yรผksek yรผzdeli avantajlฤฑ promosyonlar da ilgi รงekiyor. Bu nedenle, Casibom รงabucak piyasada iyi bir pazarlama baลarฤฑsฤฑ elde ediyor ve bรผyรผk bir oyuncularฤฑn kitlesi kazanฤฑyor.
Casibom’un kar getiren bonuslarฤฑ ve tanฤฑnฤฑrlฤฑฤฤฑ ile birlikte, web sitesine abonelik ne ลekilde saฤlanฤฑr sorusuna da bahsetmek gerekir. Casibom’a hareketli cihazlarฤฑnฤฑzdan, bilgisayarlarฤฑnฤฑzdan veya tabletlerinizden tarayฤฑcฤฑ รผzerinden rahatรงa eriลilebilir. Ayrฤฑca, sitenin mobil cihazlarla uyumlu olmasฤฑ da bรผyรผk bir avantaj sunuyor, รงรผnkรผ artฤฑk neredeyse herkesin bir cep telefonu var ve bu telefonlar รผzerinden kolayca ulaลฤฑm saฤlanabiliyor.
Hareketli cihazlarฤฑnฤฑzla bile yolda canlฤฑ iddialar alabilir ve maรงlarฤฑ canlฤฑ olarak izleyebilirsiniz. Ayrฤฑca, Casibom’un mobil uyumlu olmasฤฑ, รผlkemizde kumarhane ve oyun gibi yerlerin meลru olarak kapatฤฑlmasฤฑyla birlikte bu tรผr platformlara eriลimin bรผyรผk bir yolunu oluลturuyor.
Casibom’un emin bir kumarhane platformu olmasฤฑ da รถnemlidir bir avantaj getiriyor. Ruhsatlฤฑ bir platform olan Casibom, kesintisiz bir ลekilde keyif ve kar saฤlama imkanฤฑ sunar.
Casibom’a รผye olmak da oldukรงa kolaydฤฑr. Herhangi bir belge koลulu olmadan ve รผcret รถdemeden platforma kolayca kullanฤฑcฤฑ olabilirsiniz. Ayrฤฑca, web sitesi รผzerinde para yatฤฑrma ve รงekme iลlemleri iรงin de birรงok farklฤฑ yรถntem bulunmaktadฤฑr ve herhangi bir kesim รผcreti talep edilmemektedir.
Ancak, Casibom’un gรผncel giriล adresini takip etmek de elzemdir. รรผnkรผ canlฤฑ bahis ve casino web siteleri popรผler olduฤu iรงin hileli web siteleri ve dolandฤฑrฤฑcฤฑlar da gรถrรผnmektedir. Bu nedenle, Casibom’un sosyal medya hesaplarฤฑnฤฑ ve gรผncel giriล adresini periyodik olarak kontrol etmek รถnemlidir.
Sonuรง, Casibom hem gรผvenilir hem de kazanรง saฤlayan bir bahis sitesi olarak ilgi รงekiyor. yรผksek รถdรผlleri, geniล oyun alternatifleri ve kullanฤฑcฤฑ dostu taลฤฑnabilir uygulamasฤฑ ile Casibom, casino hayranlarฤฑ iรงin mรผkemmel bir platform saฤlar.
http://ciprofloxacin.tech/# buy cipro cheap
https://nolvadex.life/# does tamoxifen cause weight loss
buy cytotec over the counter [url=https://cytotec.club/#]cytotec abortion pill[/url] Misoprostol 200 mg buy online
lisinopril tablets for sale: compare zestril prices – lisinopril comparison
cipro for sale [url=https://ciprofloxacin.tech/#]buy ciprofloxacin over the counter[/url] ciprofloxacin 500 mg tablet price
http://nolvadex.life/# tamoxifen alternatives
https://ciprofloxacin.tech/# ciprofloxacin 500 mg tablet price
cost generic propecia without a prescription [url=http://finasteride.store/#]buy propecia price[/url] cheap propecia no prescription
order propecia no prescription: get generic propecia without dr prescription – buy generic propecia prices
https://finasteride.store/# get generic propecia prices
cipro 500mg best prices [url=https://ciprofloxacin.tech/#]ะฟยปัcipro generic[/url] buy ciprofloxacin over the counter
http://nolvadex.life/# tamoxifen 20 mg
purchase famciclovir pills – valaciclovir oral valcivir 500mg generic
lisinopril 5 mg for sale [url=http://lisinopril.network/#]buy cheap lisinopril 40mg[/url] no prescription lisinopril
nolvadex estrogen blocker: nolvadex for pct – raloxifene vs tamoxifen
๋ก๋์คํ๊ณผ์ ๋ ๋ฒ๋ฆฌ์ง ์คํ: ํฌ์๋ฒ์ ์ฐธ์ ํ ์งํ
๋ก๋์คํ์ ํตํด ์ ๊ณต๋๋ ๋ ๋ฒ๋ฆฌ์ง ๋ฐฉ์์ ์คํ์ ์ฃผ์ ํฌ์์ ํ ๋ฐฉ๋ฒ์ผ๋ก, ํฐ ์์ต๋ฅ ์ ๋ชฉํ๋ก ํ๋ ํฌ์์๋ค์๊ฒ ์ ํน์ ์ธ ์ ํ์ ๋๋ค. ๋ ๋ฒ๋ฆฌ์ง๋ฅผ ์ด์ฉํ๋ ์ด ์ ๋ต์ ํฌ์์๊ฐ ์์ ์ ํฌ์๊ธ์ ์ด๊ณผํ๋ ํฌ์๊ธ์ ํฌ์ํ ์ ์๋๋ก ํ์ฌ, ์ฆ๊ถ ์ฅ์์ ํจ์ฌ ํฐ ์ํฅ๋ ฅ์ ๊ฐ์ง ์ ์๋ ๋ฐฉ๋ฒ์ ์ค๋๋ค.
๋ ๋ฒ๋ฆฌ์ง ์คํ์ ๊ธฐ๋ณธ ์์น
๋ ๋ฒ๋ฆฌ์ง ๋ฐฉ์์ ์คํ์ ๊ธฐ๋ณธ์ ์ผ๋ก ์๋ณธ์ ๋น๋ ค ์ฌ์ฉํ๋ ๋ฐฉ์์ ๋๋ค. ์ฌ๋ก๋ฅผ ๋ค์ด, 100๋ง ์์ ํฌ์๊ธ์ผ๋ก 1,000๋ง ์ ์๋น์ ์ฆ๊ถ์ ์ฌ๋ค์ผ ์ ์๋๋ฐ, ์ด๋ ํฌ์์๋ค์ด ๊ธฐ๋ณธ์ ์ธ ์๋ณธ๋ณด๋ค ํจ์ฌ ๋ ๋ง์ ์ฃผ์์ ์ทจ๋ํ์ฌ, ์ฃผ์ ๊ฐ๊ฒฉ์ด ์์นํ ๊ฒฝ์ฐ ํด๋นํ๋ ๋์ฑ ํฐ ์ด์ต์ ์ป์ ์ ์๊ฒ ๋ฉ๋๋ค. ๊ทธ๋ ์ง๋ง, ์ฆ๊ถ ๊ฐ๊ฒฉ์ด ๋จ์ด์ง ๊ฒฝ์ฐ์๋ ๊ทธ ์ํด ๋ํ ์ฆ๊ฐํ ์ ์์ผ๋ฏ๋ก, ๋ ๋ฒ๋ฆฌ์ง ์ฌ์ฉ์ ์ฌ์ฉํ ๋๋ ์ ์คํด์ผ ํฉ๋๋ค.
ํฌ์ ๊ณํ๊ณผ ๋ ๋ฒ๋ฆฌ์ง ์ฌ์ฉ
๋ ๋ฒ๋ฆฌ์ง ์ฌ์ฉ์ ํนํ ์ฑ์ฅ ์ ์ฌ๋ ฅ์ด ํฐ ์ฌ์ ์ฒด์ ํฌ์ํ ๋ ๋์์ด ๋ฉ๋๋ค. ์ด๋ฌํ ๊ธฐ์ ์ ์๋นํ ๋น์จ์ ํตํด ํฌ์ ํ๋ฉด, ์ฑ๊ณตํ ๊ฒฝ์ฐ ๋ง๋ํ ์์ ์ ์ป์ ์ ์์ง๋ง, ๋ฐ๋์ ๊ฒฝ์ฐ ๋ง์ ์ํ๋ ์ง์ด์ ธ์ผ ํฉ๋๋ค. ๊ทธ๋ ๊ธฐ ๋๋ฌธ์, ํฌ์ํ๋ ์ฌ๋์ ์์ ์ ์ํ์ฑ ๊ด๋ฆฌ ๋ฅ๋ ฅ์ ๊ฐ์ง ์ฅํฐ ๋ถ์์ ํตํด, ์ด๋ ๊ธฐ์ ์ ์ผ๋ง๋งํผ์ ํฌ์๊ธ์ ์ ์ฉํ ์ง ์ ํํด์ผ ํฉ๋๋ค.
๋ ๋ฒ๋ฆฌ์ง์ ์ด์ ๊ณผ ์ํ ์์
๋ ๋ฒ๋ฆฌ์ง ๋ฐฉ์์ ์คํ์ ํฐ ์์ต์ ์ ๊ณตํ์ง๋ง, ๊ทธ๋งํผ ์๋นํ ์ํ๋ ์๋ฐํฉ๋๋ค. ์ฆ๊ถ ์์ฅ์ ๋ณํ๋ ์ถ์ ์ด ์ด๋ ต๊ธฐ ๋๋ฌธ์, ๋ ๋ฒ๋ฆฌ์ง ์ฌ์ฉ์ ์ฌ์ฉํ ๋๋ ์ธ์ ๋ ์ฅํฐ ์ถ์ธ๋ฅผ ์ ๋ฐํ๊ฒ ๊ด์ฐฐํ๊ณ , ์์ค์ ์ต์ํํ ์ ์๋ ๊ณํ์ ๋ง๋ จํด์ผ ํฉ๋๋ค.
์ต์ข ์ ์ผ๋ก: ์ ์คํ ์ ํ์ด ํ์์ ๋๋ค
๋ก๋์คํ์์ ์ ๊ณตํ๋ ๋ ๋ฒ๋ฆฌ์ง ๋ฐฉ์์ ์คํ์ ๋ง๊ฐํ ํฌ์ ์๋จ์ด๋ฉฐ, ์ ๋นํ ์ด์ฉํ๋ฉด ๋ง์ ์์ต์ ๊ฐ์ ธ๋ค์ค ์ ์์ต๋๋ค. ํ์ง๋ง ํฐ ์ํ์ฑ๋ ์ ๊ฒฝ ์จ์ผ ํ๋ฉฐ, ํฌ์ ๊ฒฐ์ ์ ํ์ํ ์ฌ์ค๊ณผ ์ ์คํ ํ๋จ ํ์ ์ค์๋์ด์ผ ํฉ๋๋ค. ํฌ์์ ์์ ์ ์ฌ์ ์ํฉ, ์ํ์ ๊ฐ์ํ๋ ๋ฅ๋ ฅ, ๊ทธ๋ฆฌ๊ณ ์ฅํฐ ์ํฉ์ ๊ณ ๋ คํ ์์ ๋ ํฌ์ ๊ณํ์ด ์ค์ํ๋ฉฐ.
https://finasteride.store/# generic propecia without insurance
tamoxifen dosage [url=http://nolvadex.life/#]how to prevent hair loss while on tamoxifen[/url] low dose tamoxifen
http://finasteride.store/# buying cheap propecia without insurance
buy digoxin without a prescription – purchase calan generic buy generic lasix
lisinopril 20mg tablets cost: prescription medicine lisinopril – lisinopril from canada
cheap propecia prices propecia buy buy cheap propecia tablets
cipro for sale: ciprofloxacin 500mg buy online – cipro online no prescription in the usa
cost generic propecia prices propecia without a prescription cost of propecia price
https://finasteride.store/# buying cheap propecia price
http://nolvadex.life/# tamoxifen joint pain
ciprofloxacin where can i buy cipro online cipro
buy cytotec pills: buy cytotec – buy cytotec over the counter
http://ciprofloxacin.tech/# ciprofloxacin
Backlinks seo
Effective Links in Weblogs and Comments: Increase Your SEO
Links are crucial for boosting search engine rankings and raising web site visibility. By integrating backlinks into weblogs and comments wisely, they can considerably increase targeted traffic and SEO overall performance.
Adhering to Search Engine Algorithms
The current day’s backlink placement strategies are finely tuned to align with search engine algorithms, which now prioritize link good quality and relevance. This ensures that hyperlinks are not just numerous but meaningful, directing end users to helpful and pertinent articles. Website owners should emphasis on integrating backlinks that are contextually suitable and improve the overall articles quality.
Rewards of Using Clean Contributor Bases
Utilizing up-to-date donor bases for links, like those handled by Alex, provides significant advantages. These bases are frequently refreshed and consist of unmoderated sites that donโt attract complaints, ensuring the links placed are both impactful and certified. This method will help in sustaining the efficacy of backlinks without the dangers linked with moderated or troublesome assets.
Only Sanctioned Resources
All donor sites used are approved, keeping away from legal pitfalls and sticking to digital marketing criteria. This commitment to utilizing only approved resources guarantees that each backlink is legitimate and trustworthy, thereby building reliability and dependability in your digital existence.
SEO Influence
Skillfully positioned backlinks in blogs and remarks provide over just SEO advantagesโthey boost user experience by linking to pertinent and high-quality content. This strategy not only satisfies search engine criteria but also entails end users, leading to better targeted traffic and enhanced online involvement.
In essence, the right backlink strategy, particularly one that utilizes fresh and reliable donor bases like Alexโs, can change your SEO efforts. By concentrating on quality over amount and adhering to the newest standards, you can guarantee your backlinks are both powerful and effective.
tamoxifen therapy tamoxifen and osteoporosis clomid nolvadex
tamoxifen estrogen: how to lose weight on tamoxifen – where to buy nolvadex
http://cytotec.club/# cytotec abortion pill
http://nolvadex.life/# what is tamoxifen used for
purchase cytotec cytotec abortion pill buy cytotec
get propecia without insurance: buying generic propecia without rx – order generic propecia without rx
Kantorbola situs slot online terbaik 2024 , segera daftar di situs kantor bola dan dapatkan promo terbaik bonus deposit harian 100 ribu , bonus rollingan 1% dan bonus cashback mingguan . Kunjungi juga link alternatif kami di kantorbola77 , kantorbola88 dan kantorbola99
ะัะพะฒะตัะบะฐ ะดะฐะฝะฝัั ะบะพัะตะปัะบะพะฒ ะดะปั ั ัะฐะฝะตะฝะธั ะบัะธะฟัะพะฒะฐะปััั ะฝะฐ ะฝะฐะปะธัะธะต ะฟะพะดะพะทัะธัะตะปัะฝัั ััะตะดััะฒ: ะะฑะตัะฟะตัะตะฝะธะต ะฑะตะทะพะฟะฐัะฝะพััะธ ัะฒะพะตะณะพ ะบัะธะฟัะพะฒะฐะปััะฝะพะณะพ ะฟะพัััะตะปััะธะบะฐ
ะ ะผะธัะต ัะปะตะบััะพะฝะฝัั ะดะตะฝะตะณ ััะฐะฝะพะฒะธััั ะฒัะต ะฑะพะปะตะต ัััะตััะฒะตะฝะฝะตะต ะพะฑะตัะฟะตัะธะฒะฐัั ะฑะตะทะพะฟะฐัะฝะพััั ัะฒะพะธั ัะธะฝะฐะฝัะพะฒัั ะฐะบัะธะฒะพะฒ. ะะถะตะดะฝะตะฒะฝะพ ะผะพัะตะฝะฝะธะบะธ ะธ ั ะฐะบะตัั ัะพะทะดะฐัั ัะฒะตะถะธะต ะฟะพะดั ะพะดั ะพะฑะผะฐะฝะฐ ะธ ะผะพัะตะฝะฝะธัะตััะฒะฐ ะธ ะบัะฐะถะธ ะฒะธัััะฐะปัะฝัั ะดะตะฝะตะณ. ะะดะฝะธะผ ะธะท ัััะตััะฒะตะฝะฝัั ััะตะดััะฒ ะพะฑะตัะฟะตัะตะฝะธั ะฑะตะทะพะฟะฐัะฝะพััะธ ัะฒะปัะตััั ะฟัะพะฒะตัะบะฐ ะดะฐะฝะฝัั ะบะพัะตะปัะบะฐ ะฟะพ ะฒััะฒะปะตะฝะธะต ะฝะตะปะตะณะฐะปัะฝัั ััะตะดััะฒ.
ะะพัะตะผั ะฒะพั ะฒะฐะถะฝะพ, ััะพะฑั ะฟัะพะฒะตัััั ัะพะฑััะฒะตะฝะฝัะต ัะธััะพะฒัะต ะฑัะผะฐะถะฝะธะบะธ?
ะัะตะถะดะต ะฒัะตะณะพ, ะฒะพั ััะพ ะฝัะถะฝะพ ะดะปั ะพะฑะตัะฟะตัะตะฝะธั ะฑะตะทะพะฟะฐัะฝะพััะธ ัะพะฑััะฒะตะฝะฝัั ััะตะดััะฒ. ะะฝะพะณะธะต ะฟะพะปัะทะพะฒะฐัะตะปะธ ััะฐะปะบะธะฒะฐัััั ั ัะธัะบะพะผ ะฟะพัะตัะธ ะดะตะฝะตะณ ะธั ััะตะดััะฒ ะฒัะปะตะดััะฒะธะต ะฝะตะดะพะฑัะพัะพะฒะตััะฝัั ะผะตัะพะดะพะฒ ะธะปะธ ะบัะฐะถ. ะะฝะฐะปะธะท ะบะพัะตะปัะบะพะฒ ะดะปั ั ัะฐะฝะตะฝะธั ะบัะธะฟัะพะฒะฐะปััั ะฟะพะผะพะณะฐะตั ัะฒะพะตะฒัะตะผะตะฝะฝะพ ะฒััะฒะธัั ัะพะผะฝะธัะตะปัะฝัะต ะพะฟะตัะฐัะธะธ ะธ ะฟัะตะดัะฟัะตะดะธัั.
ะงัะพ ะฟัะตะดะปะฐะณะฐะตั ะฒะฐัะตะผั ะฒะฝะธะผะฐะฝะธั ะฝะฐัะฐ ะพัะณะฐะฝะธะทะฐัะธั?
ะั ะฟัะตะดะปะฐะณะฐะตะผ ะฟะพัะปัะณั ะฟัะพะฒะตัะบะธ ะบะพัะตะปัะบะพะฒ ัะธััะพะฒัั ะบะพัะตะปัะบะพะฒ ะดะปั ั ัะฐะฝะตะฝะธั ัะปะตะบััะพะฝะฝัั ะดะตะฝะตะณ ะธ ััะฐะฝะทะฐะบัะธะน ั ะฝะฐะผะตัะตะฝะธะตะผ ะฒััะฒะปะตะฝะธั ะฝะฐัะฐะปะฐ ััะตะดััะฒ ะฟะตัะตะดะฒะธะถะตะฝะธั ะธ ะดะฐัั ะดะตัะฐะปัะฝะพะณะพ ะพััะตัะฐ ะพ ะฟัะพะฒะตัะบะต. ะคะธัะผะฐ ะฟัะตะดะพััะฐะฒะปัะตั ะฟัะพะณัะฐะผะผะฐ ะพัะผะฐััะธะฒะฐะตั ะดะฐะฝะฝัะต ะฟะพะปัะทะพะฒะฐัะตะปั ะดะปั ะฒััะฒะปะตะฝะธั ะฝะตะทะฐะบะพะฝะฝัั ะพะฟะตัะฐัะธะน ะธ ะพัะตะฝะธัั ัะธัะบ ะดะปั ัะพะณะพ, ััะพะฑั ะฒะฐัะตะณะพ ะฟะพัััะตะปั ะฐะบัะธะฒะพะฒ. ะะปะฐะณะพะดะฐัั ะฝะฐัะตะผั ะฐะฝะฐะปะธะทั, ะฒั ัะผะพะถะตัะต ะฟัะตะดะพัะฒัะฐัะธัั ั ัะตะณัะปััะพัะฐะผะธ ะธ ะทะฐัะธัะธัั ะพั ะฝะตะฟัะตะดะฝะฐะผะตัะตะฝะฝะพะณะพ ััะฐััะธั ะฒ ัะธะฝะฐะฝัะธัะพะฒะฐะฝะธะธ ะฝะตะทะฐะบะพะฝะฝัั ะดะตััะตะปัะฝะพััะตะน.
ะะฐะบ ะพัััะตััะฒะปัะตััั ะฟัะพัะตัั?
ะะพะผะฟะฐะฝะธั ะฝะฐัะฐ ะพัะณะฐะฝะธะทะฐัะธั ัะพัััะดะฝะธัะฐะตั ั ะฐะฒัะพัะธัะตัะฝัะผะธ ะฐัะดะธัะพััะบะธะผะธ ะพัะณะฐะฝะธะทะฐัะธัะผะธ ััััะบัััะฐะผะธ, ะฒัะพะดะต Certik, ะดะปั ัะพะณะพ, ััะพะฑั ะพะฑะตัะฟะตัะธัั ะธ ัะพัะฝะพััั ะฝะฐัะธั ะฟัะพะฒะตัะพะบ. ะั ะธัะฟะพะปัะทัะตะผ ัะพะฒัะตะผะตะฝะฝัะต ัะตั ะฝะพะปะพะณะธะธ ะธ ัะตั ะฝะธะบะธ ะฐะฝะฐะปะธะทะฐ ะดะฐะฝะฝัั ะดะปั ะฒััะฒะปะตะฝะธั ะฝะฐะปะธัะธั ะพะฟะฐัะฝัั ะพะฟะตัะฐัะธะน ััะตะดััะฒ. ะะตััะพะฝะฐะปัะฝัะต ะดะฐะฝะฝัะต ะฝะฐัะธั ะทะฐะบะฐะทัะธะบะพะฒ ะพะฑัะฐะฑะฐััะฒะฐัััั ะธ ั ัะฐะฝัััั ะฒ ัะฟะตัะธะฐะปัะฝะพะน ะฑะฐะทะต ะดะฐะฝะฝัั ะฒ ัะพะพัะฒะตัััะฒะธะธ ะฒััะพะบะธะผะธ ััะตะฑะพะฒะฐะฝะธัะผะธ.
ะะฐะถะฝัะน ะทะฐะฟัะพั: “ะฟัะพะฒะตัะธัั ัะฒะพะธ USDT ะฝะฐ ัะธััะพัั”
ะ ัะปััะฐะต ะตัะปะธ ะฒั ั ะพัะธัะต ัะฑะตะดะธัััั ะฑะตะทะพะฟะฐัะฝะพััะธ ะธ ัะธััะพัะต ะฒะฐัะธั USDT ะบะพัะตะปัะบะพะฒ, ะฝะฐัะธ ัะบัะฟะตััั ะพะบะฐะทัะฒะฐะตั ะฒะพะทะผะพะถะฝะพััั ะฑะตัะฟะปะฐัะฝัะน ะฐะฝะฐะปะธะท ะฟะตัะฒัั ะฟััะธ ะบะพัะตะปัะบะพะฒ. ะัะพััะพ ัะฒะพะน ะบะพัะตะปะตะบ ะฒ ะฝัะถะฝะพะต ะผะตััะพ ะฝะฐ ะฝะฐัะตะผ ะพะฝะปะฐะนะฝ-ัะตััััะต, ะธ ะผั ะฒััะปะตะผ ะฒะฐะผ ะดะตัะฐะปัะฝัะน ะพััะตั ะพ ะตะณะพ ััะฐัััะต.
ะะฑะตัะฟะตัััะต ะทะฐัะธัั ัะฒะพะธั ะฐะบัะธะฒั ะฒ ะดะฐะฝะฝัะน ะผะพะผะตะฝั!
ะัะตะดะพัะฒัะฐัะฐะนัะต ัะธัะบะธ ะพะบะฐะทะฐัััั ะฒ ะฟะพัััะฐะดะฐัั ะบัะธะผะธะฝะฐะปัะฝัั ัะปะตะผะตะฝัะพะฒ ะธะปะธ ััะฐัั ะฒ ะฝะตะฟัะธััะฝะพะน ัะธััะฐัะธะธ ะฝะตะฟัะฐะฒะพะผะตัะฝัั ัะดะตะปะพะบ ั ะฒะฐัะธะผะธ ัะธะฝะฐะฝัะฐะผะธ. ะะพะทะฒะพะปััะต ัะตะฑะต ะฟัะพัะตััะธะพะฝะฐะปะฐะผ, ะบะพัะพััะต ะฟะพะผะพะณัั, ะฒะฐะผ ะธ ะฒะฐัะธะผ ัะธะฝะฐะฝัะฐะผ ะทะฐัะธัะธัััั ะบัะธะฟัะพะฒะฐะปััะฝัะต ััะตะดััะฒะฐ ะธ ะฟัะตะดะพัะฒัะฐัะธัั ะฒะพะทะผะพะถะฝัะต. ะกะพะฒะตััะธัะต ะฟะตัะฒัะน ัะฐะณ ะบ ะฑะตะทะพะฟะฐัะฝะพััะธ ะพะฑะตัะฟะตัะตะฝะธั ะฑะตะทะพะฟะฐัะฝะพััะธ ะฒะฐัะตะณะพ ัะธััะพะฒะพะณะพ ัะธะฝะฐะฝัะพะฒะพะณะพ ะฟะพัััะตะปั ะฟััะผะพ ัะตะนัะฐั!
http://finasteride.store/# propecia no prescription
buy propecia tablets propecia sale order cheap propecia no prescription
cipro pharmacy: cipro ciprofloxacin – buy ciprofloxacin
ัะธัััะน ะปะธ usdt
ะะฝะฐะปะธะท Tether ะฒ ัะธััะพัั: ะะฐะบะพะฒัะผ ัะฟะพัะพะฑะพะผ ัะพั ัะฐะฝะธัั ัะฒะพะธ ัะธััะพะฒัะต ัะธะฝะฐะฝัั
ะะพััะพัะฝะฝะพ ะฒัะต ะฑะพะปััะต ะฟะพะปัะทะพะฒะฐัะตะปะตะน ะพะฑัะฐัะฐัั ะฒะฝะธะผะฐะฝะธะต ะบ ะฑะตะทะพะฟะฐัะฝะพััั ะปะธัะฝัั ะบัะธะฟัะพะฒะฐะปััะฝัั ะฐะบัะธะฒะพะฒ. ะะตะฝั ะพัะพ ะดะฝั ะดะตะปััั ัะฐะทัะฐะฑะฐััะฒะฐัั ะฝะพะฒัะต ะฟะพะดั ะพะดั ัะฐะทะณัะฐะฑะปะตะฝะธั ัะธััะพะฒัั ะดะตะฝะตะณ, ะธะปะธ ะฒะปะฐะดะตะปััั ะบัะธะฟัะพะฒะฐะปััั ััะฐะฝะพะฒัััั ะถะตััะฒะฐะผะธ ะธั ะพะฑะผะฐะฝะพะฒ. ะะดะธะฝ ะฟะพะดั ะพะดะพะฒ ะพั ัะฐะฝั ััะฐะฝะพะฒะธััั ัะตััะธัะพะฒะฐะฝะธะต ะบะพัะตะปัะบะพะฒ ะฝะฐ ะฟัะธัััััะฒะธะต ะฝะตะทะฐะบะพะฝะฝัั ัะธะฝะฐะฝัะพะฒ.
ะก ะบะฐะบะพะน ัะตะปัั ััะพ ะฝะตะพะฑั ะพะดะธะผะพ?
ะัะตะถะดะต ะฒัะตะณะพ, ะดะปั ัะพะณะพ ััะพะฑั ัะพั ัะฐะฝะธัั ะปะธัะฝัะต ััะตะดััะฒะฐ ะพั ัะฐัะปะฐัะฐะฝะพะฒ ะธ ะฟะพั ะธัะตะฝะฝัั ะดะตะฝะตะณ. ะะฝะพะณะธะต ะฒะบะปะฐะดัะธะบะธ ััะฐะปะบะธะฒะฐัััั ั ัะธัะบะพะผ ัััะฐัั ะธั ัะพะฝะดะพะฒ ะธะท-ะทะฐ ั ะธัะฝัั ััะตะฝะฐัะธะตะฒ ะธะปะธ ะบัะฐะถะตะน. ะัะผะพัั ะฑัะผะฐะถะฝะธะบะพะฒ ะฟะพะผะพะณะฐะตั ะฒััะฒะธัั ะฟะพะดะพะทัะธัะตะปัะฝัะต ะดะตะนััะฒะธั ะฐ ัะฐะบะถะต ะฟัะตะดะพัะฒัะฐัะธัั ะฒะพะทะผะพะถะฝัะต ะฟะพัะตัะธ.
ะงัะพ ะผั ะฟัะตะดะปะฐะณะฐะตะผ?
ะั ะฟัะตะดะพััะฐะฒะปัะตะผ ะฟะพะดั ะพะด ะฟัะพะฒะตัะบะธ ะบัะธะฟัะพะฒะฐะปััะฝัั ะฑัะผะฐะถะฝะธะบะพะฒ ะธ ััะฐะฝะทะฐะบัะธะน ะดะปั ะพะฑะฝะฐััะถะตะฝะธั ะฟัะพะธัั ะพะถะดะตะฝะธั ัะพะฝะดะพะฒ. ะะฐัะฐ ัะธััะตะผะฐ ะฐะฝะฐะปะธะทะธััะตั ะดะฐะฝะฝัะต ะดะปั ะฒััะฒะปะตะฝะธั ะฝะตะทะฐะบะพะฝะฝัั ะดะตะนััะฒะธะน ะธ ะพัะตะฝะบะธ ัะธัะบะฐ ะดะปั ะฒะฐัะตะณะพ ะฟะพัััะตะปั. ะะปะฐะณะพะดะฐัั ะดะฐะฝะฝะพะน ะฟัะพะฒะตัะบะต, ะฒั ัะผะพะถะตัะต ะธะทะฑะตะถะฐัั ะฟัะพะฑะปะตะผ ั ัะตะณัะปััะพัะฐะผะธ ะธะปะธ ะพะฑะตะทะพะฟะฐัะธัั ัะตะฑั ะพั ััะฐััะธั ะฒ ะฝะตะทะฐะบะพะฝะฝัั ะพะฟะตัะฐัะธัั .
ะะฐะบ ััะพ ัะฐะฑะพัะฐะตั?
ะั ัะพัััะดะฝะธัะฐะตะผ ั ะปัััะธะผะธ ะฟัะพะฒะตัะพัะฝัะผะธ ะบะพะผะฟะฐะฝะธัะผะธ, ะฒัะพะดะต Cure53, ะดะปั ัะพะณะพ ััะพะฑั ะฟัะตะดะพััะฐะฒะธัั ัะพัะฝะพััั ะฝะฐัะธั ัะตััะธัะพะฒะฐะฝะธะน. ะะฐัะฐ ะบะพะผะฐะฝะดะฐ ะฟัะธะผะตะฝัะตะผ ะฟะตัะตะดะพะฒัะต ัะตั ะฝะพะปะพะณะธะธ ะดะปั ะฒััะฒะปะตะฝะธั ะพะฟะฐัะฝัั ะพะฟะตัะฐัะธะน. ะะฐัะธ ะดะฐะฝะฝัะต ะฟัะพั ะพะดัั ะพะฑัะฐะฑะพัะบั ะธ ั ัะฐะฝัััั ัะพะณะปะฐัะฝะพ ั ะฒััะพะบะธะผะธ ััะฐะฝะดะฐััะฐะผะธ ะฑะตะทะพะฟะฐัะฝะพััะธ ะธ ะฟัะธะฒะฐัะฝะพััะธ.
ะะฐะบะธะผ ะพะฑัะฐะทะพะผ ะฟัะพะฒะตัะธัั ัะพะฑััะฒะตะฝะฝัะต Tether ะดะปั ะฟัะพะทัะฐัะฝะพััั?
ะัะธ ะฝะฐะปะธัะธะธ ะถะตะปะฐะฝะธั ัะฑะตะดะธัััั, ััะพ ะฒะฐัะฐ USDT-ะบะพัะตะปัะบะธ ัะธััั, ะฝะฐั ัะตัะฒะธั ะฟัะตะดะพััะฐะฒะปัะตั ะฑะตัะฟะปะฐัะฝะพะต ัะตััะธัะพะฒะฐะฝะธะต ะฟะตัะฒัั ะฟััะธ ะฑัะผะฐะถะฝะธะบะพะฒ. ะัะพััะพ ะฟะตัะตะดะฐะนัะต ะผะตััะพะฟะพะปะพะถะตะฝะธะต ัะฒะพะตะณะพ ะบะพัะตะปัะบะฐ ะฝะฐ ะฝะฐ ัะฐะนัะต, ะธ ะผั ะฟัะตะดะพััะฐะฒะธะผ ะฒะฐะผ ะฟะพะปะฝัั ะธะฝัะพัะผะฐัะธั ะพััะตั ะพะฑ ะตะณะพ ััะฐัััะต.
ะะฐัะฐะฝัะธััะนัะต ะฑะตะทะพะฟะฐัะฝะพััั ะดะปั ะฒะฐัะธะผะธ ะฐะบัะธะฒั ัะตะณะพะดะฝั ะถะต!
ะะต ะฟะพะดะฒะตัะณะฐะนัะต ะพะฟะฐัะฝะพััะธ ััะฐัั ะถะตััะฒะพะน ะดะตะปััะพะฒ ะธะปะธ ะฟะพะฟะฐะดะฐัั ะฒ ะฝะตะฟัะธััะฝัั ะพะฑััะฐะฝะพะฒะบั ะฒัะปะตะดััะฒะธะต ะฝะตะปะตะณะฐะปัะฝัั ะพะฟะตัะฐัะธะน. ะะพัะตัะธัะต ะฝะฐะผ, ััะพะฑั ะพะฑะตะทะพะฟะฐัะธัั ะฒะฐัะธ ัะธััะพะฒัะต ัะธะฝะฐะฝัะพะฒัะต ัะตััััั ะธ ะฟัะตะดะพัะฒัะฐัะธัั ัะปะพะถะฝะพััะตะน. ะกะดะตะปะฐะนัะต ะฟะตัะฒัะน ัะฐะณ ะดะปั ัะพั ัะฐะฝะฝะพััะธ ะฒะฐัะตะณะพ ะบัะธะฟัะพะฒะฐะปััะฝะพะณะพ ะฟะพัััะตะปั ะฟััะผะพ ัะตะนัะฐั!
ciprofloxacin 500 mg tablet price buy cipro online ciprofloxacin 500 mg tablet price
buy microzide 25mg generic – cost felodipine 10mg buy generic bisoprolol
https://cytotec.club/# buy cytotec online fast delivery
ะัะพะฒะตัะบะฐ Tether ะฒ ะฟัะพะทัะฐัะฝะพััั: ะะฐะบะธะผ ะพะฑัะฐะทะพะผ ะพะฑะตะทะพะฟะฐัะธัั ะปะธัะฝัะต ัะปะตะบััะพะฝะฝัะต ัะธะฝะฐะฝัั
ะัะต ะฑะพะปะตะต ะฟะพะปัะทะพะฒะฐัะตะปะตะน ะพะฑัะฐัะฐัั ะฒะฝะธะผะฐะฝะธะต ะฝะฐ ะฝะฐะดะตะถะฝะพััั ัะฒะพะธั ัะปะตะบััะพะฝะฝัั ะฐะบัะธะฒะพะฒ. ะะถะตะดะฝะตะฒะฝะพ ัะฐัะปะฐัะฐะฝั ะฟัะตะดะปะฐะณะฐัั ะฝะพะฒัะต ัั ะตะผั ะบัะฐะถะธ ะบัะธะฟัะพะฒะฐะปััะฝัั ััะตะดััะฒ, ะฐ ัะฐะบะถะต ะฒะปะฐะดะตะปััั ัะปะตะบััะพะฝะฝะพะน ะฒะฐะปััั ะพะบะฐะทัะฒะฐัััั ะฟะพัััะฐะดะฐะฒัะธะผะธ ะธั ะฟะพะดััะฐะฒ. ะะดะธะฝ ะธะท ัะฟะพัะพะฑะพะฒ ะพั ัะฐะฝั ััะฐะฝะพะฒะธััั ัะตััะธัะพะฒะฐะฝะธะต ะบะพัะตะปัะบะพะฒ ะฒ ะฝะฐะปะธัะธะต ะฝะตะปะตะณะฐะปัะฝัั ัะธะฝะฐะฝัะพะฒ.
ะก ะบะฐะบะธะผ ะฝะฐะผะตัะตะฝะธะตะผ ััะพ ะฒะฐะถะฝะพ?
ะัะตะถะดะต ะฒัะตะณะพ, ะดะปั ัะพะณะพ ััะพะฑั ะทะฐัะธัะธัั ัะฒะพะธ ััะตะดััะฒะฐ ะฟัะพัะธะฒ ัะฐัะปะฐัะฐะฝะพะฒ ะธะปะธ ะฟะพั ะธัะตะฝะฝัั ะผะพะฝะตั. ะะฝะพะณะธะต ะธะฝะฒะตััะพัั ััะฐะปะบะธะฒะฐัััั ั ัะธัะบะพะผ ะฟะพัะตัะธ ัะฒะพะธั ััะตะดััะฒ ะฒัะปะตะดััะฒะธะต ะผะพัะตะฝะฝะธัะตัะบะธั ะผะตั ะฐะฝะธะทะผะพะฒ ะปะธะฑะพ ะบัะฐะถะตะน. ะัะผะพัั ะบะพัะตะปัะบะพะฒ ะฟะพะผะพะณะฐะตั ะฒััะฒะธัั ะฝะตะฟัะพะทัะฐัะฝัะต ะพะฟะตัะฐัะธะธ ะธะปะธ ะฟัะตะดะพัะฒัะฐัะธัั ะฒะพะทะผะพะถะฝัะต ะฟะพัะตัะธ.
ะงัะพ ะฝะฐัะฐ ะณััะฟะฟะฐ ะฟัะตะดะพััะฐะฒะปัะตะผ?
ะั ะฟัะตะดะปะฐะณะฐะตะผ ัะตัะฒะธั ะฟัะพะฒะตัะบะธ ัะปะตะบััะพะฝะฝัั ะบะพัะตะปัะบะพะฒ ะฐ ัะฐะบะถะต ััะฐะฝะทะฐะบัะธะน ะดะปั ะพะฟัะตะดะตะปะตะฝะธั ะฝะฐัะฐะปะฐ ััะตะดััะฒ. ะะฐัะฐ ะฟะปะฐััะพัะผะฐ ะฟัะพะฒะตััะตั ะดะฐะฝะฝัะต ะดะปั ะพะฟัะตะดะตะปะตะฝะธั ะฟัะพัะธะฒะพะทะฐะบะพะฝะฝัั ะพะฟะตัะฐัะธะน ะธ ะฟัะพัะตะฝะบะธ ัะธัะบะฐ ะดะปั ะฒะฐัะตะณะพ ะฟะพัััะตะปั. ะะท-ะทะฐ ััะพะน ะฟัะพะฒะตัะบะต, ะฒั ัะผะพะถะตัะต ะธะทะฑะตะถะฐัั ะฟัะพะฑะปะตะผ ั ัะตะณัะปััะพัะฐะผะธ ะธะปะธ ะฟัะตะดะพั ัะฐะฝะธัั ัะตะฑั ะพั ััะฐััะธั ะฒ ะฝะตะปะตะณะฐะปัะฝัั ะพะฟะตัะฐัะธัั .
ะะฐะบะธะผ ะพะฑัะฐะทะพะผ ััะพ ัะฐะฑะพัะฐะตั?
ะะฐัะฐ ะบะพะผะฐะฝะดะฐ ัะฐะฑะพัะฐะตะผ ั ะฟะตัะตะดะพะฒัะผะธ ะฟัะพะฒะตัะพัะฝัะผะธ ะบะพะผะฟะฐะฝะธัะผะธ, ะฒัะพะดะต Halborn, ะดะปั ัะพะณะพ ััะพะฑั ะณะฐัะฐะฝัะธัะพะฒะฐัั ะฐะบะบััะฐัะฝะพััั ะฝะฐัะธั ัะตััะธัะพะฒะฐะฝะธะน. ะั ะธัะฟะพะปัะทัะตะผ ัะพะฒัะตะผะตะฝะฝัะต ัะตั ะฝะธะบะธ ะดะปั ะพะฟัะตะดะตะปะตะฝะธั ะพะฟะฐัะฝัั ะพะฟะตัะฐัะธะน. ะะฐัะธ ะธะฝัะพัะผะฐัะธั ะพะฑัะฐะฑะฐััะฒะฐัััั ะธ ัะพั ัะฐะฝััััั ัะพะณะปะฐัะฝะพ ั ะฒััะพะบะธะผะธ ะฝะพัะผะฐะผะธ ะฑะตะทะพะฟะฐัะฝะพััะธ ะธ ะบะพะฝัะธะดะตะฝัะธะฐะปัะฝะพััะธ.
ะะฐะบ ะฟัะพะฒะตัะธัั ัะฒะพะธ USDT ะฒ ัะธััะพัั?
ะัะปะธ ะฒะฐะผ ะฝัะถะฝะพ ัะฑะตะดะธัััั, ััะพ ะฒะฐัะธ Tether-ะบะพัะตะปัะบะธ ัะธััั, ะฝะฐั ัะตัะฒะธั ะฟัะตะดะปะฐะณะฐะตั ะฑะตัะฟะปะฐัะฝะพะต ัะตััะธัะพะฒะฐะฝะธะต ะฟะตัะฒัั ะฟััะธ ะฑัะผะฐะถะฝะธะบะพะฒ. ะัะพััะพ ะฒะฒะตะดะธัะต ะผะตััะพ ัะพะฑััะฒะตะฝะฝะพะณะพ ะบะพัะตะปัะบะฐ ะฝะฐ ะฝะฐ ะฝะฐัะตะผ ะฒะตะฑ-ัะฐะนัะต, ะธ ัะฐะบะถะต ะผั ะฟัะตะดะพััะฐะฒะธะผ ะฒะฐะผ ะดะตัะฐะปัะฝัะน ะพััะตั ะพะฑ ะตะณะพ ััะฐัััะต.
ะะฑะตะทะพะฟะฐัััะต ะฒะฐัะธ ะฐะบัะธะฒั ะฟััะผะพ ัะตะนัะฐั!
ะะทะฑะตะณะฐะนัะต ัะธัะบะฐ ะฟะพะดะฒะตัะณะฝััััั ะพะฑะผะฐะฝัะธะบะพะฒ ะธะปะธ ะพะบะฐะทะฐัััั ะฒ ะฝะตะฟัะธััะฝัั ะพะฑััะฐะฝะพะฒะบั ะฒัะปะตะดััะฒะธะต ะฝะตะทะฐะบะพะฝะฝัั ะพะฟะตัะฐัะธะน. ะะฑัะฐัะธัะตัั ะทะฐ ะฟะพะผะพััั ะบ ะฝะฐัะตะผั ะฐะณะตะฝัััะฒั, ะดะปั ัะพะณะพ ััะพะฑั ัะพั ัะฐะฝะธัั ะฒะฐัะธ ัะธััะพะฒัะต ัะธะฝะฐะฝัะพะฒัะต ัะตััััั ะธ ะธะทะฑะตะถะฐัั ะฝะตะฟัะธััะฝะพััะตะน. ะัะธะผะธัะต ะฟะตัะฒัะน ัะฐะณ ะดะปั ะฑะตะทะพะฟะฐัะฝะพััะธ ะฒะฐัะตะณะพ ะบัะธะฟัะพะฒะฐะปััะฝะพะณะพ ะฟะพัััะตะปั ัะถะต ัะตะณะพะดะฝั!
ะขะตััะธัะพะฒะฐะฝะธะต ะขะตัะตั ะฒ ัะธััะพัั: ะะฐะบะพะฒัะผ ัะฟะพัะพะฑะพะผ ัะพั ัะฐะฝะธัั ะปะธัะฝัะต ัะธััะพะฒัะต ะฐะบัะธะฒั
ะะพััะพัะฝะฝะพ ะฒัะต ะฑะพะปััะต ะปัะดะตะน ะพะฑัะฐัะฐัั ะฒะฝะธะผะฐะฝะธะต ะดะปั ะฑะตะทะพะฟะฐัะฝะพััั ัะพะฑััะฒะตะฝะฝัั ะบัะธะฟัะพะฒะฐะปััะฝัั ะฐะบัะธะฒะพะฒ. ะะตะฝั ะพัะพ ะดะฝั ะดะตะปััั ะฟัะธะดัะผัะฒะฐัั ะฝะพะฒัะต ะผะตัะพะดั ะบัะฐะถะธ ัะปะตะบััะพะฝะฝัั ััะตะดััะฒ, ะฐ ัะฐะบะถะต ัะพะฑััะฒะตะฝะฝะธะบะธ ัะธััะพะฒะพะน ะฒะฐะปััั ะพะบะฐะทัะฒะฐัััั ะถะตััะฒะฐะผะธ ะธั ะพะฑะผะฐะฝะพะฒ. ะะดะธะฝ ัะฟะพัะพะฑะพะฒ ัะฑะตัะตะถะตะฝะธั ััะฐะฝะพะฒะธััั ัะตััะธัะพะฒะฐะฝะธะต ะบะพัะตะปัะบะพะฒ ะดะปั ะฟัะธัััััะฒะธะต ะฝะตะปะตะณะฐะปัะฝัั ััะตะดััะฒ.
ะก ะบะฐะบะพะน ัะตะปัั ััะพ ะฟะพะปะตะทะฝะพ?
ะัะตะถะดะต ะฒัะตะณะพ, ััะพะฑั ะพะฑะตะทะพะฟะฐัะธัั ะปะธัะฝัะต ะฐะบัะธะฒั ะฟัะพัะธะฒ ะดะตะปััะพะฒ ะฐ ัะฐะบะถะต ะฟะพั ะธัะตะฝะฝัั ะผะพะฝะตั. ะะฝะพะณะธะต ััะฐััะฝะธะบะธ ััะฐะปะบะธะฒะฐัััั ั ะฟะพัะตะฝัะธะฐะปัะฝะพะน ัะณัะพะทะพะน ัะฑััะบะพะฒ ะธั ัะธะฝะฐะฝัะพะฒ ะฒ ัะตะทัะปััะฐัะต ะพะฑะผะฐะฝะฝัั ััะตะฝะฐัะธะตะฒ ะธะปะธ ะบัะฐะถะตะน. ะัะผะพัั ะฑัะผะฐะถะฝะธะบะพะฒ ะฟะพะผะพะณะฐะตั ะฒััะฒะธัั ะฝะตะฟัะพะทัะฐัะฝัะต ะดะตะนััะฒะธั ะฐ ัะฐะบะถะต ะฟัะตะดะพัะฒัะฐัะธัั ะฒะพะทะผะพะถะฝัะต ะฟะพัะตัะธ.
ะงัะพ ะผั ะฟัะตะดะปะฐะณะฐะตะผ?
ะั ะฟัะตะดะปะฐะณะฐะตะผ ััะปัะณั ัะตััะธัะพะฒะฐะฝะธั ะบัะธะฟัะพะฒะฐะปััะฝัั ะฑัะผะฐะถะฝะธะบะพะฒ ะฐ ัะฐะบะถะต ััะฐะฝะทะฐะบัะธะน ะดะปั ะพะฟัะตะดะตะปะตะฝะธั ะฝะฐัะฐะปะฐ ััะตะดััะฒ. ะะฐัะฐ ัะธััะตะผะฐ ะฐะฝะฐะปะธะทะธััะตั ะธะฝัะพัะผะฐัะธั ะดะปั ะฒััะฒะปะตะฝะธั ะฝะตะทะฐะบะพะฝะฝัั ะพะฟะตัะฐัะธะน ะธะปะธ ะพัะตะฝะบะธ ัะณัะพะทั ะฒะฐัะตะณะพ ััะตัะฐ. ะะปะฐะณะพะดะฐัั ัะฐะบะพะน ะฟัะพะฒะตัะบะต, ะฒั ัะผะพะถะตัะต ะธะทะฑะตะณะฐัั ะฟัะพะฑะปะตะผ ั ัะตะณัะปััะพัะฐะผะธ ะธ ะพะฑะตะทะพะฟะฐัะธัั ัะตะฑั ะพั ััะฐััะธั ะฒ ะฝะตะทะฐะบะพะฝะฝัั ะฟะตัะตะฒะพะดะฐั .
ะะฐะบ ะฟัะพะธัั ะพะดะธั ะฟัะพัะตัั?
ะะฐัะฐ ัะธัะผะฐ ัะพัััะดะฝะธัะฐะตะผ ั ะฟะตัะตะดะพะฒัะผะธ ะฟัะพะฒะตัะพัะฝัะผะธ ะบะพะผะฟะฐะฝะธัะผะธ, ะฝะฐะฟัะธะผะตั Certik, ััะพะฑั ะฟัะตะดะพััะฐะฒะธัั ัะพัะฝะพััั ะฝะฐัะธั ะฟัะพะฒะตัะพะบ. ะั ะฟัะธะผะตะฝัะตะผ ะฝะพะฒะตะนัะธะต ัะตั ะฝะธะบะธ ะดะปั ะพะฑะฝะฐััะถะตะฝะธั ัะธัะบะพะฒะฐะฝะฝัั ะพะฟะตัะฐัะธะน. ะะฐัะธ ะดะฐะฝะฝัะต ะพะฑัะฐะฑะฐััะฒะฐัััั ะธ ั ัะฐะฝัััั ัะพะณะปะฐัะฝะพ ั ะฒััะพะบะธะผะธ ะฝะพัะผะฐะผะธ ะฑะตะทะพะฟะฐัะฝะพััะธ ะธ ะบะพะฝัะธะดะตะฝัะธะฐะปัะฝะพััะธ.
ะะฐะบะธะผ ะพะฑัะฐะทะพะผ ะฟัะพะฒะตัะธัั ัะฒะพะธ USDT ะฝะฐ ะฝะตััะพะฝััะพััั?
ะัะปะธ ะฒะฐะผ ะฝัะถะฝะพ ัะฑะตะดะธัััั, ััะพ ะฒะฐัะฐ Tether-ะบะพัะตะปัะบะธ ะฝะตััะพะฝััั, ะฝะฐั ัะตัะฒะธั ะฟัะตะดะพััะฐะฒะปัะตั ะฑะตัะฟะปะฐัะฝะพะต ัะตััะธัะพะฒะฐะฝะธะต ะฟะตัะฒัั ะฟััะธ ะฑัะผะฐะถะฝะธะบะพะฒ. ะัะพััะพ ะฒะฑะตะนัะต ะฐะดัะตั ะปะธัะฝะพะณะพ ะบะพัะตะปัะบะฐ ะฝะฐ ะฝะฐ ัะฐะนัะต, ะธะปะธ ะฝะฐั ัะตัะฒะธั ะฟัะตะดะปะพะถะธะผ ะฒะฐะผ ะฟะพะดัะพะฑะฝัะน ะดะพะบะปะฐะด ะพ ะตะณะพ ะฟะพะปะพะถะตะฝะธะธ.
ะะฑะตะทะพะฟะฐัััะต ะฒะฐัะธะผะธ ะฐะบัะธะฒั ัะถะต ัะตะนัะฐั!
ะะต ะฟะพะดะฒะตัะณะฐะนัะต ะพะฟะฐัะฝะพััะธ ััะฐัั ะถะตััะฒะพะน ัะฐัะปะฐัะฐะฝะพะฒ ะธะปะธ ะฟะพะฟะฐััั ะฒ ะฝะตะฑะปะฐะณะพะฟัะธััะฝัั ะพะฑััะฐะฝะพะฒะบั ะธะท-ะทะฐ ะฟัะพัะธะฒะพะทะฐะบะพะฝะฝัั ะพะฟะตัะฐัะธะน. ะะฑัะฐัะธัะตัั ะทะฐ ะฟะพะผะพััั ะบ ะฝะฐัะตะน ะบะพะผะฐะฝะดะต, ั ัะตะผ ััะพะฑั ะทะฐัะธัะธัั ะฒะฐัะธ ะบัะธะฟัะพะฒะฐะปััะฝัะต ัะธะฝะฐะฝัะพะฒัะต ัะตััััั ะธ ะธะทะฑะตะถะฐัั ะฟัะพะฑะปะตะผ. ะัะตะดะฟัะธะผะธัะต ะฟะตัะฒัะน ัะฐะณ ะดะปั ะฑะตะทะพะฟะฐัะฝะพััะธ ะฒะฐัะตะณะพ ะบัะธะฟัะพะฒะฐะปััะฝะพะณะพ ะฟะพัััะตะปั ัะถะต ัะตะณะพะดะฝั!
kantor bola
Situs kantor bola merupakan penyedia permainan slot online gacor dengan RTP 98% , mainkan game slot online mudah menang di situs kantorbola . tersedia fitur deposit kilat menggunakan QRIS Kantorbola .
ะะฐะบ ัะฑะตะดะธัััั ะฒ ัะธััะพัะต USDT
ะัะพะฒะตัะบะฐ ะดะฐะฝะฝัั ะฑัะผะฐะถะฝะธะบะพะฒ ะทะฐ ะฟัะธัััััะฒะธะต ะฝะตะปะตะณะฐะปัะฝัั ัะธะฝะฐะฝัะพะฒัั ััะตะดััะฒ: ะะฐัะธัะฐ ัะฒะพะตะณะพ ะบัะธะฟัะพะฒะฐะปััะฝะพะณะพ ัะธะฝะฐะฝัะพะฒะพะณะพ ะฟะพัััะตะปั
ะ ะผะธัะต ัะปะตะบััะพะฝะฝัั ะดะตะฝะตะณ ััะฐะฝะพะฒะธััั ะฒัะต ะฑะพะปะตะต ะฒะฐะถะฝะตะต ะพะฑะตัะฟะตัะธะฒะฐัั ะฑะตะทะพะฟะฐัะฝะพััั ัะพะฑััะฒะตะฝะฝัั ะดะตะฝะตะณ. ะะพััะพัะฝะฝะพ ะผะพัะตะฝะฝะธะบะธ ะธ ะบะธะฑะตัะฟัะตัััะฟะฝะธะบะธ ัะฐะทัะฐะฑะฐััะฒะฐัั ัะฒะตะถะธะต ัั ะตะผั ะพะฑะผะฐะฝะฐ ะธ ะฒะพัะพะฒััะฒะฐ ะฒะธัััะฐะปัะฝัั ัะธะฝะฐะฝัะพะฒ. ะะดะธะฝ ะธะท ะพัะฝะพะฒะฝัั ะธะฝััััะผะตะฝัะพะฒ ะพะฑะตัะฟะตัะตะฝะธั ััะฐะฝะพะฒะธััั ะฐะฝะฐะปะธะท ะบะพัะตะปัะบะพะฒ ะฝะฐ ะฒััะฒะปะตะฝะธะต ะฝะตะทะฐะบะพะฝะฝัั ััะตะดััะฒ.
ะะพัะตะผั ะถะต ัะฐะบ ะฒะฐะถะฝะพ ะธ ะฟัะพะฒะตัััั ัะพะฑััะฒะตะฝะฝัะต ัะปะตะบััะพะฝะฝัะต ะบะพัะตะปัะบะธ ะดะปั ั ัะฐะฝะตะฝะธั ะบัะธะฟัะพะฒะฐะปััั?
ะ ะฟะตัะฒัั ะพัะตัะตะดั, ะฒะพั ััะพั ะผะพะผะตะฝั ะฝะตะพะฑั ะพะดะธะผะพ ะดะปั ะพั ัะฐะฝั ัะพะฑััะฒะตะฝะฝัั ัะธะฝะฐะฝัะพะฒัั ััะตะดััะฒ. ะะพะปััะธะฝััะฒะพ ะปัะดะธ, ะฒะบะปะฐะดัะฒะฐััะธะต ะดะตะฝัะณะธ ัะธัะบััั ะฟะพัะตัััั ะฟะพัะตัะธ ะดะตะฝะตะณ ะธั ัะธะฝะฐะฝัะพะฒัั ััะตะดััะฒ ะฒ ัะตะทัะปััะฐัะต ะฝะตัะฟัะฐะฒะตะดะปะธะฒัั ะฟะพะดั ะพะดะพะฒ ะธะปะธ ัะณะพะฝะพะฒ. ะัะพะฒะตัะบะฐ ะดะฐะฝะฝัั ะฑัะผะฐะถะฝะธะบะพะฒ ะฟะพะผะพะณะฐะตั ะพะฑะฝะฐััะถะธัั ะฒ ะฝัะถะฝัะน ะผะพะผะตะฝั ะฟะพะดะพะทัะธัะตะปัะฝัะต ะดะตะนััะฒะธั ะธ ะฟัะตะดะพัะฒัะฐัะธัั ะฒะพะทะผะพะถะฝัะต ัะฑััะบะธ.
ะงัะพ ะฟัะตะดะพััะฐะฒะปัะตั ะบะพะผะฟะฐะฝะธั?
ะั ะฟัะตะดะพััะฐะฒะปัะตะผ ัะตัะฒะธั ะฟัะพะฒะตัะบะธ ะฟัะพะฒะตัะบะธ ะบัะธะฟัะพะฒะฐะปััะฝัั ะบะพัะตะปัะบะพะฒ ะดะปั ั ัะฐะฝะตะฝะธั ะบัะธะฟัะพะฒะฐะปััั ะธ ะฟะตัะตะฒะพะดะพะฒ ััะตะดััะฒ ั ะฝะฐะผะตัะตะฝะธะตะผ ะธะดะตะฝัะธัะธะบะฐัะธะธ ะฟัะพะธัั ะพะถะดะตะฝะธั ะดะตะฝะตะณ ะธ ะฟัะตะดะพััะฐะฒะปะตะฝะธั ะฟะพะปะฝะพะณะพ ะพััะตัะฐ ะพ ัะตะทัะปััะฐัะฐั . ะคะธัะผะฐ ะฟัะตะดะพััะฐะฒะปัะตั ัะธััะตะผะฐ ะฟัะพะฐะฝะฐะปะธะทะธัะพะฒะฐัั ะดะฐะฝะฝัะต ะดะปั ะฒััะฒะปะตะฝะธั ะฝะตะฟัะฐะฒะพะผะตัะฝัั ะพะฟะตัะฐัะธะน ััะตะดััะฒ ะธ ะพะฟัะตะดะตะปะธัั ััะพะฒะตะฝั ัะธัะบะฐ ะดะปั ัะพะณะพ, ััะพะฑั ัะฒะพะตะณะพ ะบัะธะฟัะพะฒะฐะปััะฝะพะณะพ ะฟะพัััะตะปั. ะะปะฐะณะพะดะฐัั ะฝะฐัะตะน ัะปัะถะฑะต ะฟัะพะฒะตัะบะธ, ะฒั ัะผะพะถะตัะต ะฟัะตะดะพัะฒัะฐัะธัั ะฒะพะทะผะพะถะฝัะต ะฟัะพะฑะปะตะผั ั ัะตะณัะปััะพัะฐะผะธ ะธ ะทะฐัะธัะธัั ะพั ัะปััะฐะนะฝะพะน ะฒะพะฒะปะตัะตะฝะฝะพััะธ ะฒ ะฝะตะทะฐะบะพะฝะฝัั ะดะตะนััะฒะธะน.
ะะฐะบ ะฟัะพะธัั ะพะดะธั ะฟัะพัะตัั?
ะะฐัะฐ ะบะพะผะฟะฐะฝะธั ะธะผะตะตั ะดะตะปะพ ั ะบััะฟะฝัะผะธ ะฐัะดะธัะพัะฐะผะธ ะพัะณะฐะฝะธะทะฐัะธัะผะธ, ะฒัะพะดะต Halborn, ััะพะฑั ะพะฑะตัะฟะตัะธัั ะธ ะฐะดะตะบะฒะฐัะฝะพััั ะฝะฐัะธั ะฟัะพะฒะตัะพะบ ะดะฐะฝะฝัั . ะั ะธัะฟะพะปัะทัะตะผ ัะพะฒัะตะผะตะฝะฝัะต ัะตั ะฝะพะปะพะณะธะธ ะธ ะผะตัะพะดะธะบะธ ะฐะฝะฐะปะธะทะฐ ะดะฐะฝะฝัั ะดะปั ะธะดะตะฝัะธัะธะบะฐัะธะธ ะพะฟะฐัะฝัั ะผะฐะฝะธะฟัะปััะธะน. ะะตััะพะฝะฐะปัะฝัะต ะดะฐะฝะฝัะต ะฝะฐัะธั ะฟะพะปัะทะพะฒะฐัะตะปะตะน ะพะฑัะฐะฑะฐััะฒะฐัััั ะธ ั ัะฐะฝัััั ะฒ ัะฟะตัะธะฐะปัะฝะพะน ะฑะฐะทะต ะดะฐะฝะฝัั ะฒ ัะพะพัะฒะตัััะฒะธะธ ั ะฒััะพะบะธะผะธ ััะฐะฝะดะฐััะฐะผะธ ะฑะตะทะพะฟะฐัะฝะพััะธ.
ะัะฝะพะฒะฝะฐั ะฟัะพััะฑะฐ: “ะฟัะพะฒะตัะธัั ัะฒะพะธ USDT ะฝะฐ ัะธััะพัั”
ะัะปะธ ะฒะฐั ะธะฝัะตัะตััะตั ัะฑะตะดะธัััั ะฑะตะทะพะฟะฐัะฝะพััะธ ะปะธัะฝัั USDT ะบะพัะตะปัะบะพะฒ, ะฝะฐัะธ ัะฟะตัะธะฐะปะธััั ะพะบะฐะทัะฒะฐะตั ัะฐะฝั ะฑะตัะฟะปะฐัะฝัะน ะฐะฝะฐะปะธะท ะฟะตัะฒัั ะฟััะธ ะบะพัะตะปัะบะพะฒ. ะัะพััะพ ัะฒะพะน ะบะพัะตะปะตะบ ะฒ ะฝัะถะฝะพะต ะผะตััะพ ะฝะฐ ะฝะฐัะตะผ ะฒะตะฑ-ัะฐะนัะต, ะธ ะผั ะฒััะปะตะผ ะฒะฐะผ ะฟะพะดัะพะฑะฝัะน ะพััะตั ะพ ะตะณะพ ััะฐัััะต.
ะะฑะตัะฟะตัััะต ะฑะตะทะพะฟะฐัะฝะพััั ัะฒะพะธั ัะธะฝะฐะฝัะพะฒัะต ััะตะดััะฒะฐ ะฒ ะดะฐะฝะฝัะน ะผะพะผะตะฝั!
ะะทะฑะตะณะฐะนัะต ัะธัะบะฐ ะฟะพะฟะฐััั ะฟะพัััะฐะดะฐัั ั ะฐะบะตัะพะฒ ะธะปะธ ะฟะพะฟะฐััั ะฝะตะฟัะธััะฝะพะผ ะฟะพะปะพะถะตะฝะธะธ ะฝะตะฟัะฐะฒะพะผะตัะฝัั ะพะฟะตัะฐัะธะน ั ะฒะฐัะธะผะธ ะปะธัะฝัะผะธ ัะธะฝะฐะฝัะฐะผะธ. ะะพะทะฒะพะปััะต ัะตะฑะต ัะฟะตัะธะฐะปะธััะฐะผ, ะบะพัะพััะต ะฟะพะผะพะณัั, ะฒะฐะผ ะพะฑะตะทะพะฟะฐัะธัััั ะบัะธะฟัะพะฒะฐะปััะฝัะต ััะตะดััะฒะฐ ะธ ะฟัะตะดะพัะฒัะฐัะธัั ะฒะพะทะผะพะถะฝัะต. ะกะดะตะปะฐะนัะต ะฟะตัะฒัะน ัะฐะณ ะบ ะทะฐัะธัะต ะฑะตะทะพะฟะฐัะฝะพััะธ ะปะธัะฝะพะณะพ ะบัะธะฟัะพะฒะฐะปััะฝะพะณะพ ะฟะพัััะตะปััะธะบะฐ ัะถะต ัะตะณะพะดะฝั!
https://ciprofloxacin.tech/# buy generic ciprofloxacin
lisinopril 420: lisinopril 10mg price in india – lisinopril 2018
Misoprostol 200 mg buy online buy cytotec cytotec pills buy online
http://ciprofloxacin.tech/# buy cipro online
cialis otc usa
ะขะตัะตั – ัะฒะปัะตััั ัััะพะนัะธะฒะฐั ะบัะธะฟัะพะฒะฐะปััะฝัะน ะฐะบัะธะฒ, ะฟัะธะฒัะทะฐะฝะฝะฐั ะบ ัะธะฐัะฝะพะน ะฒะฐะปััะต, ะฝะฐะฟัะธะผะตั USD. ะญัะพ ะฟะพะทะฒะพะปัะตั ะตะต ะฒ ัะฐััะฝะพััะธ ะฟะพะฟัะปััะฝะพะน ััะตะดะธ ะธะฝะฒะตััะพัะพะฒ, ะฟะพัะบะพะปัะบั ะดะฐะฝะฝัะน ะฐะบัะธะฒ ะฟัะตะดะพััะฐะฒะปัะตั ััะฐะฑะธะปัะฝะพััั ัะตะฝั ะฒ ะฒ ััะปะพะฒะธัั ะฝะตัััะพะนัะธะฒะพััะธ ััะฝะบะฐ ัะธััะพะฒัั ะฐะบัะธะฒะพะฒ. ะัะต ะถะต, ัะฐะบะถะต ะบะฐะบ ะธ ะปัะฑะฐั ะดััะณะฐั ะฒะธะด ัะธััะพะฒัั ะฐะบัะธะฒะพะฒ, USDT ะฟะพะดะฒะตัะถะตะฝะฐ ัะธัะบั ะธัะฟะพะปัะทะพะฒะฐะฝะธั ะดะปั ัะบัััะธั ะฟัะพะธัั ะพะถะดะตะฝะธั ััะตะดััะฒ ะธ ะฟะพะดะดะตัะถะบะธ ะฟัะพัะธะฒะพะฟัะฐะฒะฝัั ะพะฟะตัะฐัะธะน.
ะะตะณะฐะปะธะทะฐัะธั ะดะพั ะพะดะพะฒ ะฟััะตะผ ะบัะธะฟัะพะฒะฐะปััั ััะฐะฝะพะฒะธััั ะฒัะต ะฑะพะปะตะต ัะธัะพะบะพ ัะฐัะฟัะพัััะฐะฝะตะฝะฝัะผ ะฟััะตะผ ั ัะตะปัั ัะพะบัััะธั ะฟัะพะธัั ะพะถะดะตะฝะธั ะบะฐะฟะธัะฐะปะฐ. ะัะฟะพะปัะทัั ัะฐะทะฝัะต ะผะตัะพะดั, ะดะตะปััั ะผะพะณัั ััะฐัะฐัััั ะฟัะพะผัะฒะฐัั ะฝะตะทะฐะบะพะฝะฝะพ ะฟะพะปััะตะฝะฝัะต ัะพะฝะดั ะฟะพััะตะดััะฒะพะผ ัะตัะฒะธัั ะพะฑะผะตะฝะฐ ะบัะธะฟัะพะฒะฐะปัั ะธะปะธ ะผะธะบัะตัั, ะดะปั ัะพะณะพ ััะพะฑั ะพัััะตััะฒะธัั ะฟัะพะธัั ะพะถะดะตะฝะธะต ะผะตะฝะตะต ะฟะพะฝััะฝัะผ.
ะะผะตะฝะฝะพ ะดะปั ััะพะน ัะตะปะธ, ัะบัะฟะตััะธะทะฐ USDT ะฝะฐ ัะธััะพัั ะพะบะฐะทัะฒะฐะตััั ะฒะตััะผะฐ ัััะตััะฒะตะฝะฝะพะน ะผะตัะพะน ะฟัะตะดะพััะตัะตะถะตะฝะธั ะดะปั ัะพะณะพ ััะพะฑั ะฟะพะปัะทะพะฒะฐัะตะปะตะน ัะธััะพะฒัั ะฒะฐะปัั. ะะพัััะฟะฝั ัะฟะตัะธะฐะปะธะทะธัะพะฒะฐะฝะฝัะต ััะปัะณะธ, ะบะฐะบะธะต ะฒัะฟะพะปะฝััั ะฐะฝะฐะปะธะท ััะฐะฝะทะฐะบัะธะน ะธ ะบะพัะตะปัะบะพะฒ, ััะพะฑั ะธะดะตะฝัะธัะธัะธัะพะฒะฐัั ัะพะผะฝะธัะตะปัะฝัะต ััะฐะฝะทะฐะบัะธะธ ะธ ะฝะตะปะตะณะฐะปัะฝัะต ะธััะพัะฝะธะบะธ ััะตะดััะฒ. ะะฐะฝะฝัะต ััะปัะณะธ ัะพะดะตะนััะฒััั ะฟะพะปัะทะพะฒะฐัะตะปัะผ ะธะทะฑะตะถะฐัั ะฝะตะฟัะตะดะฝะฐะผะตัะตะฝะฝะพะณะพ ััะฐััะธั ะฒ ัะธะฝะฐะฝัะธัะพะฒะฐะฝะธะต ะฟัะตัััะฟะฝัั ะฐะบัะธะน ะธ ะธะทะฑะตะถะฐัั ะฑะปะพะบะธัะพะฒะบะธ ััะตัะพะฒ ัะพ ััะพัะพะฝั ะบะพะฝััะพะปะธััััะธั ะพัะณะฐะฝะพะฒ.
ะะฝะฐะปะธะท USDT ะฝะฐ ัะธััะพัั ัะฐะบะถะต ัะฐะบะถะต ะฟะพะผะพะณะฐะตั ะพะฑะตะทะพะฟะฐัะธัั ัะตะฑั ะพั ะฟะพัะตะฝัะธะฐะปัะฝัั ัะธะฝะฐะฝัะพะฒัั ะฟะพัะตัั. ะฃัะฐััะฝะธะบะธ ะผะพะณัั ะฑััั ัะฒะตัะตะฝะฝั ะฒ ัะพะผ, ััะพ ะธั ะฐะบัะธะฒั ะฝะต ะฐััะพัะธะธัะพะฒะฐะฝั ั ะฝะตะปะตะณะฐะปัะฝัะผะธ ััะฐะฝะทะฐะบัะธัะผะธ, ััะพ ะฒ ัะฒะพั ะพัะตัะตะดั ัะผะตะฝััะฐะตั ะฒะตัะพััะฝะพััั ะฑะปะพะบะธัะพะฒะบะธ ััะตัะฐ ะธะปะธ ะฟะตัะตัะธัะปะตะฝะธั ะดะตะฝะตะณ.
ะขะฐะบะธะผ ะพะฑัะฐะทะพะผ, ะฒ ััะปะพะฒะธัั ัะพะฒัะตะผะตะฝะฝะพััะธ ัะฐััััะตะน ัะปะพะถะฝะพััะธ ััะตะดั ะบัะธะฟัะพะฒะฐะปัั ะฝะตะพะฑั ะพะดะธะผะพ ะฟัะธะฝะธะผะฐัั ะผะตัั ะดะปั ะพะฑะตัะฟะตัะตะฝะธั ะฝะฐะดะตะถะฝะพััะธ ัะฒะพะธั ัะธะฝะฐะฝัะพะฒัั ัะตััััะพะฒ. ะะฝะฐะปะธะท USDT ะฝะฐ ัะธััะพัั ะฟัะธ ะฟะพะผะพัะธ ัะฟะตัะธะฐะปัะฝัั ััะปัะณ ััะฐะฝะพะฒะธััั ะพะดะฝะธะผ ะธะท ะฒะฐัะธะฐะฝัะพะฒ ะฟัะพัะธะฒะพะดะตะนััะฒะธั ัะธะฝะฐะฝัะธัะพะฒะฐะฝะธั ะฟัะตัััะฟะฝะพะน ะดะตััะตะปัะฝะพััะธ, ะฟัะตะดะพััะฐะฒะปัั ะฟะพะปัะทะพะฒะฐัะตะปัะผ ะบัะธะฟัะพะฒะฐะปัั ะดะพะฟะพะปะฝะธัะตะปัะฝัั ะทะฐัะธัั ะธ ะทะฐัะธัั.
ะัะพะฒะตัะบะฐ USDT ะฝะฐ ัะธััะพัั
ะขะตััะธัะพะฒะฐะฝะธะต USDT ะฝะฐ ัะธััะพัั: ะะฐะบะพะฒัะผ ัะฟะพัะพะฑะพะผ ะพะฑะตะทะพะฟะฐัะธัั ัะฒะพะธ ะบัะธะฟัะพะฒะฐะปััะฝัะต ััะตะดััะฒะฐ
ะะฐะถะดัะน ะดะตะฝั ะฒัะต ะฑะพะปััะต ะปัะดะตะน ะพะฑัะฐัะฐัั ะฒะฝะธะผะฐะฝะธะต ะฝะฐ ัะตะบััะธัะธ ัะฒะพะธั ะบัะธะฟัะพะฒะฐะปััะฝัั ะฐะบัะธะฒะพะฒ. ะะพััะพัะฝะฝะพ ัะฐัะปะฐัะฐะฝั ะฟัะธะดัะผัะฒะฐัั ะฝะพะฒัะต ะผะตัะพะดั ะบัะฐะถะธ ัะธััะพะฒัั ะดะตะฝะตะณ, ะธ ัะฐะบะถะต ัะพะฑััะฒะตะฝะฝะธะบะธ ะบัะธะฟัะพะฒะฐะปััั ัะฒะปััััั ะฟะพัััะฐะดะฐะฒัะธะผะธ ัะฒะพะธั ะธะฝััะธะณ. ะะดะธะฝ ะธะท ัะฟะพัะพะฑะพะฒ ัะฑะตัะตะถะตะฝะธั ััะฐะฝะพะฒะธััั ะฟัะพะฒะตัะบะฐ ะบะพัะตะปัะบะพะฒ ะฝะฐ ะฟัะธัััััะฒะธะต ะฝะตะทะฐะบะพะฝะฝัั ะดะตะฝะตะณ.
ะก ะบะฐะบะธะผ ะฝะฐะผะตัะตะฝะธะตะผ ััะพ ะฝะตะพะฑั ะพะดะธะผะพ?
ะัะตะธะผััะตััะฒะตะฝะฝะพ, ั ัะตะผ ััะพะฑั ะพะฑะตะทะพะฟะฐัะธัั ัะฒะพะธ ะฐะบัะธะฒั ะพั ัะฐัะปะฐัะฐะฝะพะฒ ะฐ ัะฐะบะถะต ะฟะพั ะธัะตะฝะฝัั ะผะพะฝะตั. ะะฝะพะณะธะต ััะฐััะฝะธะบะธ ะฒัััะตัะฐัััั ั ะฟะพัะตะฝัะธะฐะปัะฝะพะน ัะณัะพะทะพะน ัะฑััะบะพะฒ ัะฒะพะธั ัะธะฝะฐะฝัะพะฒ ะฒัะปะตะดััะฒะธะต ั ะธัะฝัั ััะตะฝะฐัะธะตะฒ ะปะธะฑะพ ะบัะฐะถะตะน. ะขะตััะธัะพะฒะฐะฝะธะต ะบะพัะตะปัะบะพะฒ ัะฟะพัะพะฑััะฒัะตั ะฒััะฒะธัั ะฝะตะฟัะพะทัะฐัะฝัะต ะพะฟะตัะฐัะธะธ ะธะปะธ ะฟัะตะดะพัะฒัะฐัะธัั ะฟะพัะตะฝัะธะฐะปัะฝัะต ะฟะพัะตัะธ.
ะงัะพ ะผั ะฟัะตะดะปะฐะณะฐะตะผ?
ะั ะฟัะตะดะพััะฐะฒะปัะตะผ ะฟะพะดั ะพะด ะฟัะพะฒะตัะบะธ ะบัะธะฟัะพะฒะฐะปััะฝัั ะฑัะผะฐะถะฝะธะบะพะฒ ะธะปะธ ะพะฟะตัะฐัะธะน ะดะปั ะพะฟัะตะดะตะปะตะฝะธั ะฝะฐัะฐะปะฐ ัะพะฝะดะพะฒ. ะะฐัะฐ ะฟะปะฐััะพัะผะฐ ะฐะฝะฐะปะธะทะธััะตั ะดะฐะฝะฝัะต ะดะปั ะพะฑะฝะฐััะถะตะฝะธั ะฟัะพัะธะฒะพะทะฐะบะพะฝะฝัั ะดะตะนััะฒะธะน ะธะปะธ ะฟัะพัะตะฝะบะธ ัะณัะพะทั ะดะปั ะฒะฐัะตะณะพ ะฟะพัััะตะปั. ะะฐ ััะตั ััะพะน ะฟัะพะฒะตัะบะต, ะฒั ัะผะพะถะตัะต ะธะทะฑะตะณะฝััั ะฝะตะดะพัะตัะพะฒ ั ัะตะณัะปััะพัะฐะผะธ ะธ ัะฐะบะถะต ะพะฑะตะทะพะฟะฐัะธัั ัะตะฑั ะพั ััะฐััะธั ะฒ ะฝะตะทะฐะบะพะฝะฝัั ะพะฟะตัะฐัะธัั .
ะะฐะบ ััะพ ะดะตะนััะฒัะตั?
ะะฐัะฐ ัะธัะผะฐ ัะพัััะดะฝะธัะฐะตะผ ั ะปัััะธะผะธ ะฐัะดะธัะพััะบะธะผะธ ะบะพะผะฟะฐะฝะธัะผะธ, ะฝะฐะฟะพะดะพะฑะธะต Halborn, ะดะปั ัะพะณะพ ััะพะฑั ะณะฐัะฐะฝัะธัะพะฒะฐัั ะฐะบะบััะฐัะฝะพััั ะฝะฐัะธั ะฟัะพะฒะตัะพะบ. ะั ะธัะฟะพะปัะทัะตะผ ัะพะฒัะตะผะตะฝะฝัะต ัะตั ะฝะพะปะพะณะธะธ ะดะปั ะพะฑะฝะฐััะถะตะฝะธั ะพะฟะฐัะฝัั ััะฐะฝะทะฐะบัะธะน. ะะฐัะธ ะดะฐะฝะฝัะต ะพะฑัะฐะฑะฐััะฒะฐัััั ะธ ัะพั ัะฐะฝััััั ัะพะณะปะฐัะฝะพ ั ะฒััะพะบะธะผะธ ะฝะพัะผะฐะผะธ ะฑะตะทะพะฟะฐัะฝะพััะธ ะธ ะฟัะธะฒะฐัะฝะพััะธ.
ะะฐะบ ะฒััะฒะธัั ัะพะฑััะฒะตะฝะฝัะต USDT ะฒ ะฟัะพะทัะฐัะฝะพััั?
ะัะปะธ ั ะพัะธัะต ัะฑะตะดะธัััั, ััะพ ะฒะฐัะฐ USDT-ะบะพัะตะปัะบะธ ะฟัะพะทัะฐัะฝั, ะฝะฐั ัะตัะฒะธั ะฟัะตะดะพััะฐะฒะปัะตั ะฑะตัะฟะปะฐัะฝัั ะฟัะพะฒะตัะบั ะฟะตัะฒัั ะฟััะธ ะบะพัะตะปัะบะพะฒ. ะัะพััะพ ะฒะฒะตะดะธัะต ะฐะดัะตั ะปะธัะฝะพะณะพ ะฑัะผะฐะถะฝะธะบะฐ ะฝะฐ ะฝะฐัะตะผ ัะฐะนัะต, ะฐ ัะฐะบะถะต ะฝะฐัะฐ ะบะพะผะฐะฝะดะฐ ะฟัะตะดะปะพะถะธะผ ะฒะฐะผ ะดะตัะฐะปัะฝัะน ะดะพะบะปะฐะด ะพ ะตะณะพ ััะฐัััะต.
ะะฐัะฐะฝัะธััะนัะต ะฑะตะทะพะฟะฐัะฝะพััั ะดะปั ะฒะฐัะธะผะธ ัะพะฝะดั ัะถะต ัะตะนัะฐั!
ะะต ะฟะพะดะฒะตัะณะฐะนัะต ะพะฟะฐัะฝะพััะธ ะฟะพะดะฒะตัะณะฝััััั ะพะฑะผะฐะฝัะธะบะพะฒ ะธะปะธ ะพะบะฐะทะฐัััั ะฒ ะฝะตะฟัะธััะฝัั ะพะฑััะฐะฝะพะฒะบั ะฒัะปะตะดััะฒะธะต ะฝะตะทะฐะบะพะฝะฝัั ัะดะตะปะพะบ. ะะฑัะฐัะธัะตัั ะบ ะฝะฐัะตะน ะบะพะผะฐะฝะดะต, ะดะปั ัะพะณะพ ััะพะฑั ะพะฑะตะทะพะฟะฐัะธัั ัะฒะพะธ ะบัะธะฟัะพะฒะฐะปััะฝัะต ะฐะบัะธะฒั ะธ ะธะทะฑะตะถะฐัั ะฝะตะฟัะธััะฝะพััะตะน. ะัะตะดะฟัะธะผะธัะต ะฟะตัะฒัะน ัะฐะณ ะบ ัะพั ัะฐะฝะฝะพััะธ ะบัะธะฟัะพะฒะฐะปััะฝะพะณะพ ะฟะพัััะตะปั ัะตะณะพะดะฝั!
ะัะผะพัั USDT ะฒ ะฟัะพะทัะฐัะฝะพััั: ะะฐะบ ะทะฐัะธัะธัั ะปะธัะฝัะต ัะปะตะบััะพะฝะฝัะต ััะตะดััะฒะฐ
ะะฐะถะดัะน ะดะตะฝั ะฒัะต ะฑะพะปััะต ะฟะพะปัะทะพะฒะฐัะตะปะตะน ะฟัะธะดะฐัั ะฒะฐะถะฝะพััั ะฒ ัะตะบััะธัะธ ัะพะฑััะฒะตะฝะฝัั ัะธััะพะฒัั ัะธะฝะฐะฝัะพะฒ. ะะพััะพัะฝะฝะพ ัะฐัะปะฐัะฐะฝั ะฟัะตะดะปะฐะณะฐัั ะฝะพะฒัะต ะฟะพะดั ะพะดั ั ะธัะตะฝะธั ัะธััะพะฒัั ะดะตะฝะตะณ, ะธ ะฒะปะฐะดะตะปััั ะบัะธะฟัะพะฒะฐะปััั ัะฒะปััััั ะฟะพัััะฐะดะฐะฒัะธะผะธ ัะฒะพะธั ะฐัะตั. ะะดะธะฝ ะธะท ะฟะพะดั ะพะดะพะฒ ะทะฐัะธัั ััะฐะฝะพะฒะธััั ะฟัะพะฒะตัะบะฐ ะฑัะผะฐะถะฝะธะบะพะฒ ะฒ ะฟัะธัััััะฒะธะต ะฟัะพัะธะฒะพะทะฐะบะพะฝะฝัั ััะตะดััะฒ.
ะก ะบะฐะบะธะผ ะฝะฐะผะตัะตะฝะธะตะผ ััะพ ะฟะพััะตะฑัะตััั?
ะัะตะถะดะต ะฒัะตะณะพ, ััะพะฑั ะทะฐัะธัะธัั ัะพะฑััะฒะตะฝะฝัะต ััะตะดััะฒะฐ ะฟัะพัะธะฒ ะผะพัะตะฝะฝะธะบะพะฒ ะธะปะธ ัะบัะฐะดะตะฝะฝัั ะผะพะฝะตั. ะะฝะพะณะธะต ัะฟะตัะธะฐะปะธััั ััะฐะปะบะธะฒะฐัััั ั ะฒะตัะพััะฝะพัััั ัััะฐัั ัะฒะพะธั ัะธะฝะฐะฝัะพะฒ ะฒ ัะตะทัะปััะฐัะต ั ะธัะฝัั ะผะตั ะฐะฝะธะทะผะพะฒ ะธะปะธ ะบัะฐะถ. ะขะตััะธัะพะฒะฐะฝะธะต ะบะพัะตะปัะบะพะฒ ะฟะพะทะฒะพะปัะตั ะฒััะฒะธัั ะฟะพะดะพะทัะธัะตะปัะฝัะต ะพะฟะตัะฐัะธะธ ะธ ัะฐะบะถะต ะฟัะตะดะพัะฒัะฐัะธัั ะฒะพะทะผะพะถะฝัะต ัะฑััะบะธ.
ะงัะพ ะผั ะฟัะตะดะปะฐะณะฐะตะผ?
ะั ะฟัะตะดะพััะฐะฒะปัะตะผ ะฟะพะดั ะพะด ะฟัะพะฒะตัะบะธ ะบัะธะฟัะพะฒะฐะปััะฝัั ะบะพัะตะปัะบะพะฒ ะธ ััะฐะฝะทะฐะบัะธะน ะดะปั ะพะฑะฝะฐััะถะตะฝะธั ะฟัะพะธัั ะพะถะดะตะฝะธั ััะตะดััะฒ. ะะฐัะฐ ัะธััะตะผะฐ ะฐะฝะฐะปะธะทะธััะตั ะดะฐะฝะฝัะต ะดะปั ะฒััะฒะปะตะฝะธั ะฝะตะปะตะณะฐะปัะฝัั ััะฐะฝะทะฐะบัะธะน ะธะปะธ ะพัะตะฝะบะธ ัะณัะพะทั ะฒะฐัะตะณะพ ะฟะพัััะตะปั. ะัะปะตะดััะฒะธะต ะดะฐะฝะฝะพะน ะฟัะพะฒะตัะบะต, ะฒั ัะผะพะถะตัะต ะธะทะฑะตะณะฝััั ะฝะตะดะพัะตัะพะฒ ั ัะตะณัะปััะพัะฐะผะธ ะธ ัะฐะบะถะต ะทะฐัะธัะธัั ัะตะฑั ะพั ััะฐััะธั ะฒ ะฟัะพัะธะฒะพะทะฐะบะพะฝะฝัั ะพะฟะตัะฐัะธัั .
ะะฐะบะธะผ ะพะฑัะฐะทะพะผ ััะพ ัะฐะฑะพัะฐะตั?
ะะฐัะฐ ะบะพะผะฐะฝะดะฐ ัะพัััะดะฝะธัะฐะตะผ ั ะฟะตัะตะดะพะฒัะผะธ ะฐัะดะธัะพััะบะธะผะธ ะพัะณะฐะฝะธะทะฐัะธัะผะธ, ะฝะฐะฟัะธะผะตั Cure53, ะดะปั ัะพะณะพ ััะพะฑั ะพะฑะตัะฟะตัะธัั ะฐะบะบััะฐัะฝะพััั ะฝะฐัะธั ะฟัะพะฒะตัะพะบ. ะั ะธัะฟะพะปัะทัะตะผ ัะพะฒัะตะผะตะฝะฝัะต ัะตั ะฝะพะปะพะณะธะธ ะดะปั ะพะฑะฝะฐััะถะตะฝะธั ัะธัะบะพะฒะฐะฝะฝัั ะพะฟะตัะฐัะธะน. ะะฐัะธ ะดะฐะฝะฝัะต ะพะฑัะฐะฑะฐััะฒะฐัััั ะธ ั ัะฐะฝัััั ะฒ ัะพะพัะฒะตัััะฒะธะธ ั ะฒััะพะบะธะผะธ ะฝะพัะผะฐะผะธ ะฑะตะทะพะฟะฐัะฝะพััะธ ะธ ะบะพะฝัะธะดะตะฝัะธะฐะปัะฝะพััะธ.
ะะฐะบ ะฟัะพะฒะตัะธัั ัะฒะพะธ Tether ะฒ ะฟัะพะทัะฐัะฝะพััั?
ะัะปะธ ั ะพัะธัะต ะฟะพะดัะฒะตัะดะธัั, ััะพ ะฒะฐัะธ USDT-ะฑัะผะฐะถะฝะธะบะธ ะฟัะพะทัะฐัะฝั, ะฝะฐั ัะตัะฒะธั ะฟัะตะดะพััะฐะฒะปัะตั ะฑะตัะฟะปะฐัะฝะพะต ัะตััะธัะพะฒะฐะฝะธะต ะฟะตัะฒัั ะฟััะธ ะฑัะผะฐะถะฝะธะบะพะฒ. ะัะพััะพ ะฒะฒะตะดะธัะต ะผะตััะพะฟะพะปะพะถะตะฝะธะต ัะพะฑััะฒะตะฝะฝะพะณะพ ะบะพัะตะปัะบะฐ ะฒ ะฝะฐัะตะผ ัะฐะนัะต, ะฐ ัะฐะบะถะต ะฝะฐัะฐ ะบะพะผะฐะฝะดะฐ ะฟัะตะดะปะพะถะธะผ ะฒะฐะผ ะฟะพะปะฝัั ะธะฝัะพัะผะฐัะธั ะดะพะบะปะฐะด ะพ ะตะณะพ ะฟะพะปะพะถะตะฝะธะธ.
ะะฐัะฐะฝัะธััะนัะต ะฑะตะทะพะฟะฐัะฝะพััั ะดะปั ะฒะฐัะธ ััะตะดััะฒะฐ ะฟััะผะพ ัะตะนัะฐั!
ะะต ะฟะพะดะฒะตัะณะฐะนัะต ัะธัะบั ะฟะพะดะฒะตัะณะฝััััั ัะฐัะปะฐัะฐะฝะพะฒ ะธะปะธ ะฟะพะฟะฐััั ะฒ ะฝะตะฑะปะฐะณะพะฟัะธััะฝัั ัะธััะฐัะธั ะธะท-ะทะฐ ะฝะตะปะตะณะฐะปัะฝัั ััะฐะฝะทะฐะบัะธะน. ะะฑัะฐัะธัะตัั ะทะฐ ะฟะพะผะพััั ะบ ะฝะฐัะตะน ะบะพะผะฐะฝะดะต, ั ัะตะผ ััะพะฑั ะพะฑะตะทะพะฟะฐัะธัั ะฒะฐัะธ ะบัะธะฟัะพะฒะฐะปััะฝัะต ัะธะฝะฐะฝัะพะฒัะต ัะตััััั ะธ ะธะทะฑะตะถะฐัั ะทะฐัััะดะฝะตะฝะธะน. ะกะดะตะปะฐะนัะต ะฟะตัะฒัะน ัะฐะณ ะดะปั ัะพั ัะฐะฝะฝะพััะธ ะฒะฐัะตะณะพ ะบัะธะฟัะพะฒะฐะปััะฝะพะณะพ ะฟะพัััะตะปั ัะถะต ัะตะณะพะดะฝั!
lopressor for sale online – inderal 20mg oral buy nifedipine 10mg without prescription
ไนๅทๅจๆจๅ
Levitra tablet price Cheap Levitra online Buy Levitra 20mg online
what does cialis cost
http://cenforce.pro/# Cenforce 100mg tablets for sale
Cenforce 150 mg online: cenforce for sale – buy cenforce
buy cenforce: Cenforce 150 mg online – buy cenforce
buy kamagra online usa kamagra oral jelly Kamagra 100mg price
https://levitrav.store/# Buy Vardenafil online
Kamagra 100mg kamagra.win buy Kamagra
Buy Tadalafil 5mg: Generic Cialis without a doctor prescription – Buy Tadalafil 20mg
where can i buy levitra
https://rg777.app/cup-c1-202324/
Howdy! Would you mind if I share your blog with my facebook group? There’s a lot of folks that I think would really enjoy your content. Please let me know. Thank you
http://kamagra.win/# Kamagra 100mg price
Vardenafil buy online Levitra 20mg price Levitra 10 mg buy online
http://levitrav.store/# Cheap Levitra online
Viagra without a doctor prescription Canada: viagra canada – Viagra online price
Viagra tablet online: viagras.online – Generic Viagra for sale
ืงื ืืืืก ืืืจืื: ืืืืจืืฉ ืืืงืืฃ ืืงื ืืืช ืฉืจืฃ ืืืืฆืขืืช ืืืฉืืื
ืฉืจืฃ ืื ืืืืช ืื ืืชืจ ืจืฉืื ืืืืข ืืืืจืืื ืืงื ืืืช ืงื ืืืืกืื ืขื ืืื ืืืืฉืืืื ืืืืืืื ืืืืืจืื.
ืืืชืจ ืจืฉืื ืืกืคืง ืืช ืืื ืืืืืข ืืงืืฉืืจืืช ืืืืืืขืื ืืขืืื ื ืืืงืืืฆืืช ืืขืจืืฆืื ืืืืืืฆืื ืืืืืฆืื ืืืฉืงืื ืงื ืืืืก ืืืืืืจืื ืืืืื ื.
ืืื ืื, ืืืชืจ ืืฆืืขื ืืืจืื ืืคืืจื ืืืื ืืืชืืจืื ืืืืฆืขืืช ืืืฉืจืฃ ืืืงื ื ืงื ืืืืกืื ืื ืืืืช ืืืืืืจืืช ืจืื.
ืืขืืจืช ืืืืจืืืช, ืืฃ ืืฉืชืืฉืื ืืืฉืื ืืืืื ืืืืื ืก ืืืืขืจืืช ืืงื ืืืืก ืืืืืืจืื ืืคื ื ืืืืื ืืฉืืืืฉ ืืืืืืืืช ืืฉืืืืฉ.
ืืืจืืืืืื ืฉื ืืงื ืืืืก ืืืคืฉืจ ืืืฉืชืืฉืื ืืืืฆืข ืคืขืืื ืฉืื ืืช ืืฆืืขืื ืืืช ืืืื ืจืืืฉืช ืฉืจืฃ, ืงืืืช ืืืืขื ืกืืืข, ืืืืงืช ืืืืกืคืช ืืืงืืจืืช ืขื ืืืืฆืจืื. ืื ืืืช ืืืจื ื ืืื ืืฉืืืืฉ ืืคืฉืืื ืืจื ืืืคืืืงืฆืื.
ืืืฉืจ ืืืฉืจ ืืืืืจ ืืฉืืืืช ื ืืชืฉืืื, ืืืืจืืก ืืฉืชืืฉืช ืืฉืืืืช ืืืืจืืช ืืืื ืืืืื, ืืจืืืกื ืืืฉืจืื ืฉื ืืจืืืกื ืืฉืจืื ืืืืืข ืงืจืืคืืืืจืคื. ืืฉืื ืืืฆืืื ืื ืงืืื ืืืืืง ืืืืืื ืืช ืืืืื ืืืช ืืืืืงืื ืืืงืืืืื ืืืจืฅ ืฉืื ืืืคื ื ืืืฆืืข ืจืืืฉื.
ืืืืืจืื ืืฆืืข ืืชืจืื ืืช ืืฉืืขืืชืืื ืืจืืืืื ืืืื ืคืจืืืืช ืืืืืืืช ืืืืืจืื ืืืื, ืืชืงืฉืืจืช ืืืืจื ืืืืืฉืืช ืืืืื. ืื ืืกืฃ, ืืื ืืืคืฉืจ ืื ืืกื ืืืืืืืืกืื ืขืืืืืช ืจืืื ืืืื ืืืฆืืข ืืืืื ืฉื ืชืืื ืืช ืืืืืืืช.
ืืืกืืืื, ืืืืจืืก ืืืืื ืื ืื ืืืงืื ืืืืืืืื ืืืืฆืื ืืช ืื ืืืืข ืืืงืืฉืืจืืช ืืืฉืงืื ืคืจืื ืงื ืืืืก ืืคื ื ืืืืจื, ืืืืืื ืื ืืื ืืจื ืืืืืจื.
ะฟยปัkamagra kamagra.win super kamagra
https://cenforce.pro/# cheapest cenforce
http://cenforce.pro/# Buy Cenforce 100mg Online
cheapest generic levitra
Fantastic site A lot of helpful info here Im sending it to some buddies ans additionally sharing in delicious And naturally thanks on your sweat
nitroglycerin cheap – oral diovan valsartan over the counter
generic sildenafil: Cheapest place to buy Viagra – Buy Viagra online cheap
ืืืืืจืื ืืจืฉืช ืื ืืืืืืช ืืจืืฉืช ืืคืืคืืืจื ืืืืชืจ ืืขืืื ืืืงืืื, ืฉืืืืจืช ืืืืืื ืื ืื ืฉืื ืืื
ืื ืจืืื ืืขืืื. ืืืืืืจืื ืืืงืืื ืื ืืชืจืืฉืื ืืืชืื ื ืืืจืืขืื ืกืคืืจืืืืืื, ืชืืฆืืืช ืคืืืืืืืช ืืืคืืื ืชืืฆืืืช ืืื ืืืืืืจ ืื ืืฉืืื ื ืืกืคืื. ืืชืจื ื ืืืืืจืื ืืืืืจืืืืืืื ืืงืจืืืื ืืช ืื ืฉืืขืื ืืื ืืืืจ ืขื ืชืืฆืืืช ืืคืฉืจืืืช ืืืืื ืืช ืืืืืืช ืืืืืืืืช ืืืจืชืงืืช.
ืืืืืืจืื ืืืงืืื ืื ืื ืื ืืืจ ืืืง ืืฉืื ืืชืจืืืช ืืืืจื ืืืื ืจื ืืืืื ืื ืืืจ ืื ืจืง ืืืง ืืจืืื ืืืคืขืืืืช ืืชืจืืืชืืช ืืืืืืืืช, ืืื ืืื ืื ืืกืคืงืื ืจืืืืื ืืืืืืื. ืืฉืื ืฉืื ื ืืืฉืื ืืืืื ืื ืืืื ืืฉืืืืฉ, ืื ืืืคืฉืจืื ืืืืื ืืืืฉืืง ืืืื ืฆืื ืจืืขื ืขืกืงื ืื ืืฆืืื ืืื ืืื ืืืื ืืงืื.
ืืื ืืืืืื ืืืืืืจืื ืืคืื ืืืืืช ืืื ืืืืืืืช ืืื ืคืืฆืืช. ืืืืืื ืื ืื ืฉืื ืืื ืื ืจืืื ืืขืืื ืืฉืชืชืคืื ืืืืืืจืื, ืืืืืื ืกืืืื ืฉืื ืื ืฉื ืืืืืจืื. ืืืืืจืื ืืงืืื ืื ืืฆืืขืื ืืืฉืชืชืคืื ืืืืื ืืืืืืืช ืืืจืชืงืช, ืืืืคืฉืจืช ืืื ืืืื ืืช ืืคืขืืืืช ืคืืคืืืจืืช ืื ืืื ืืื ืืืื ืืงืื.
ืืื ืื ื ืืชืจ ืืชื ืืืื ืืื? ืืฆืืจืฃ ืขืืฉืื ืืืชืืื ืืืื ืืช ืืืชืจืืฉืืช ืืืื ืื ืฉืืืืืืจืื ืืืื ืืจื ื ืืืืืืื.
Kamagra 100mg kamagra.win ะฟยปัkamagra
https://cenforce.pro/# Buy Cenforce 100mg Online
cenforce.pro: Cenforce 150 mg online – Cenforce 150 mg online
cenforce.pro Cenforce 150 mg online cenforce.pro
http://levitrav.store/# Buy Vardenafil 20mg
Buy Cialis online: cialist.pro – Buy Tadalafil 20mg
ะฟยปัlegitimate online pharmacies india indian pharmacy paypal reputable indian online pharmacy
https://pharmmexico.online/# mexican online pharmacies prescription drugs
Backlink pyramid
Sure, here’s the text with spin syntax applied:
Backlink Structure
After numerous updates to the G search engine, it is required to utilize different methods for ranking.
Today there is a approach to draw the interest of search engines to your site with the aid of incoming links.
Backlinks are not only an effective marketing resource but they also have natural traffic, straight sales from these resources perhaps will not be, but click-throughs will be, and it is beneficial visitors that we also receive.
What in the end we get at the final outcome:
We present search engines site through links.
Prluuchayut organic transitions to the site and it is also a signal to search engines that the resource is used by individuals.
How we show search engines that the site is profitable:
Backlinks do to the main page where the main information.
We make backlinks through redirections reliable sites.
The most CRUCIAL we place the site on sites analyzers separate tool, the site goes into the cache of these analysis tools, then the obtained links we place as redirects on weblogs, discussion boards, comment sections. This significant action shows search engines the MAP OF THE SITE as analyzer sites show all information about sites with all key terms and headings and it is very GOOD.
All details about our services is on the website!
http://pharmnoprescription.icu/# no prescription needed pharmacy
reliable canadian pharmacy: canadian pharmacy king – canadian pharmacies that deliver to the us
buying prescription drugs online without a prescription: medications online without prescription – prescription online canada
canadian pharmacy ltd: canadian drugs pharmacy – reputable canadian online pharmacies
how to buy prescriptions from canada safely online pharmacy without prescriptions buying prescription drugs in canada